We want our websites to be responsive and provide a good browsing experience to users with different devices and screen sizes. To make this happen, we must also resize the media content, like videos, to fit seamlessly into the layout.
Most of the time, the videos that we display need to be resized to a smaller size and with a specific aspect ratio, like vertical for mobile devices (having an aspect ratio of 9:16), horizontal like different video players (16:9) or square (1:1). We often have to crop the videos to fit these dimensions and aspect ratios to ensure the main subject of the video is in focus and your web page is still optimal and engaging.
This blog will explore how to resize and crop videos directly in the browser (client-side) and discuss the challenges of this approach. We’ll then look at how to handle resizing and cropping using FFmpeg on the backend, addressing its complexities. Finally, we’ll introduce a more efficient solution using ImageKit, demonstrating how to integrate it into any React application in just minutes.
Cropping and resizing videos on the browser
Using the height
and width
property on HTML <video> element
A simple solution to resize the video that comes to mind is to use the height
and width
attributes in the <video>
element. We can set these attributes based on the viewport dimensions and add CSS breakpoints to adjust in a responsive layout. For example, if we have a video with dimensions 2160 x 3840 and size 24.3 MB, and we want to display it in our application with a width of 270 CSS pixels, we can do it using the following example:
<video width="270" controls>
<source src="https://ik.imagekit.io/<IMAGEKIT-ID>/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4" type="video/mp4" />
</video>
The browser will display the resized video. However, it must still download the large video file from the source URL. So, whether you display a larger or smaller-dimension video, the browser downloads the original video before rendering the resized video.
Moreover, setting the width and height of the video element adjusts the playback window rather than cropping or resizing the video. As a result, the video will scale to fit within the specified dimensions, preserving its original proportions. This outcome is not what we want if the goal is to resize and crop the video, such as converting it to a vertical aspect ratio dimension commonly seen in short video content. Simply resizing the player does not crop or resize the video itself.
Cropping and resizing videos on the server side
Videos should be transformed, optimised, and delivered from the server-side to be easily used in the <video>
element for faster load times and reliable playback across various devices. By handling these transformations on the server, you can deliver videos in the optimal quality, size, and format tailored to varying network conditions and device requirements. This ensures that your frontend React application always displays the right videos based on the above needs.
FFmpeg can be used for video transformations like resizing, cropping, etc. It is a preferred tool for server-side video processing because it handles various video transformations easily.
Using FFmpeg for video transformations
FFmpeg is a powerful, open-source multimedia framework used for a wide range of video and audio processing tasks. We will now look at some examples of resizing, cropping, and transcoding videos using FFmpeg’s command line tool.
Resizing video:
Resizing a video is a common operation and can be easily done using FFmpeg. To resize a video to a resolution of 1280×720 pixels, we can use this command:
ffmpeg -i input.mp4 -vf scale=1280:720 output.mp4
This command takes a video input.mp4
, applies the scale
filter to adjust the resolution, and outputs the resized video as output.mp4
.
Cropping video:
We can also crop a video to extract a specific area. For example, to crop a video from the center, say a 640×480 section, we can use this command:
ffmpeg -i input.mp4 -vf "crop=640:480:(in_w-640)/2:(in_h-480)/2" output.mp4
This command calculates the center of the video by subtracting the crop width and height from the input video dimensions (in_w
and in_h
) and dividing by 2, resulting in a centered 640×480 crop, which is then saved as output.mp4
.
Converting MP4 video to WebM:
Converting an MP4 video to the WebM format using the VP9 codec can reduce file size while keeping the quality at a similar level. Here's a simple FFmpeg command to do that:
ffmpeg -i input.mp4 -c:v libvpx-vp9 output.webm
The command above takes input.mp4
as the source video, and encodes it with the VP9 codec. The final output is saved as output.webm
.
Videos generated using these FFmpeg commands can then be stored and served to any React application.
Using FFmpeg on the browser
For scenarios like resizing or cropping videos before upload, simple in-browser editing, or basic on-the-fly transformations, a popular option is ffmpeg.wasm, a WebAssembly (WASM) port of FFmpeg. It allows you to run many of the same FFmpeg commands you would on a server, but directly in the browser. However, because it’s running client-side, it can be quite resource-intensive—using significant memory and CPU—and might lead to sluggish performance on lower-end devices. Longer initialization and processing times also become a concern when dealing with larger files or complex commands.
If you need more efficient, real-time performance—especially for interactive editing scenarios where low latency is important—the WebCodecs API is a compelling alternative. It provides low-level, hardware-accelerated video encoding and decoding, giving direct access to raw video frames. This hardware acceleration can significantly speed up tasks like resizing or cropping while reducing CPU overhead compared to software-based solutions like ffmpeg.wasm. However, keep in mind that the WebCodecs API can be more complex to implement, given its lower-level approach and current browser support constraints.
Key components for transforming and delivering videos
Let us understand what is needed to deliver these transformed videos to the frontend:
- Storage: The main video and all its resized, cropped, or transformed versions need to be stored along with any video-related metadata associated with it that we may need. We should also be able to upload, manage, and delete those videos.
- Processing: A pipeline that would take the original input video, resize, crop, and transform it to meet the requirements of our application. Videos are bigger in size compared to images, so it takes more time to process and be ready. Keeping the transformations processed before adding them to your application is suitable.
- Delivery: Different devices support different video formats. Many support the webm format, which can deliver a similar quality of video at a smaller size compared to a format like mp4. Moreover, we need to ensure that the quality of the transformed video is suitable for viewing and not degraded.
- Caching: Once any video is resized or cropped, it is prudent to store and cache it so that the same version can be picked from the cache and delivered, saving you redundant processing costs. This also provides optimal performance to your React application as the videos will be available quickly.
We will need to set up a backend application that can transform the videos to implement all this. However, there is a lot more than cropping and resizing when setting up this logic.
Even though we didn't originally set out to address these issues, delivering the video in the optimal format, and size, and with the necessary transformations remains essential.
Thankfully, we don’t have to tackle this by building our own solution to resize and crop videos in our React application. ImageKit solves all these problems and much more with its easy-to-use Video API.
Cropping and resizing videos using ImageKit
ImageKit is a real-time media processing platform that helps you process, optimise and deliver media files at scale.
Getting started
- Create a free account.
- Upload a video to the Media Library.
- Crop and resize videos using simple URL-based transformation parameters.
- Configure the imagekitio-react SDK in your project and deliver optimised cropped and resized videos.
Upload a video to the Media library
There are several ways to upload files in the Media Library. It can be done programmatically using APIs and SDKs and also from the dashboard.
Once logged in to the dashboard, navigate to the media library and click the new button to select and upload a file. You can also drag and drop a file to upload to the media library.
You can download this video for the following demonstrations:
https://ik.imagekit.io/ikmedia/blog-feb-25/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4?tr=orig

Once the video is uploaded, you can copy and paste the URL into the browser. Your file is now accessible via the URL.
The structure of a typical URL is like this:
https://ik.imagekit.io/<IMAGEKIT-ID>/<PATH-TO-YOUR-FILE>
Crop and resize using URL transformation parameters
We will use the width (w), height (h), and aspect ratio (ar) transformations to generate square, horizontal, and vertical variations of this video.
ImageKit uses URL path or query parameters to apply these transformations to the video. A path transformation parameter starts with a tr:
prefix, and the different transformation parameters are comma-separated.
Square:
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:ar-1-1,w-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
The above transformation tr:ar-1-1,w-300
creates a resized video with aspect ratio 1:1 and width 300 px.
Vertical:
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:ar-9-16,w-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
The above transformation tr:ar-9-16,w-300
creates a resized video with aspect ratio 9:16 and width 300 px.
Horizontal:
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:ar-16-9,w-800/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
The above transformation tr:ar-16-9,w-800
creates a resized video with aspect ratio 16:9 and width 800 px.
We have now resized the video horizontally, but notice that quite a bit of the main subject in the video is getting cropped. We can use various crop and crop mode transformations for a suitable output.
Pad resize cropping with blurred background in the horizontal video:
Look at the following example that uses a padding resize cropping strategy. This does not clip the video and adds padding to the leftover space on the left and right sides to respect the requested dimensions.
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:h-450,w-800,cm-pad_resize,bg-blurred/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
Thumbnail:
Another important aspect of video playback in any React application is providing a thumbnail. It improves user engagement and is essential to convey information to the user so that they can click and play it.
Using ImageKit, we can easily create thumbnails for videos using URL-based transformation on the same video file. We need to add a suffix /ik-thumbnail.jpg
to the video URL.
https://ik.imagekit.io/<IMAGEKIT-ID>/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4/ik-thumbnail.jpg
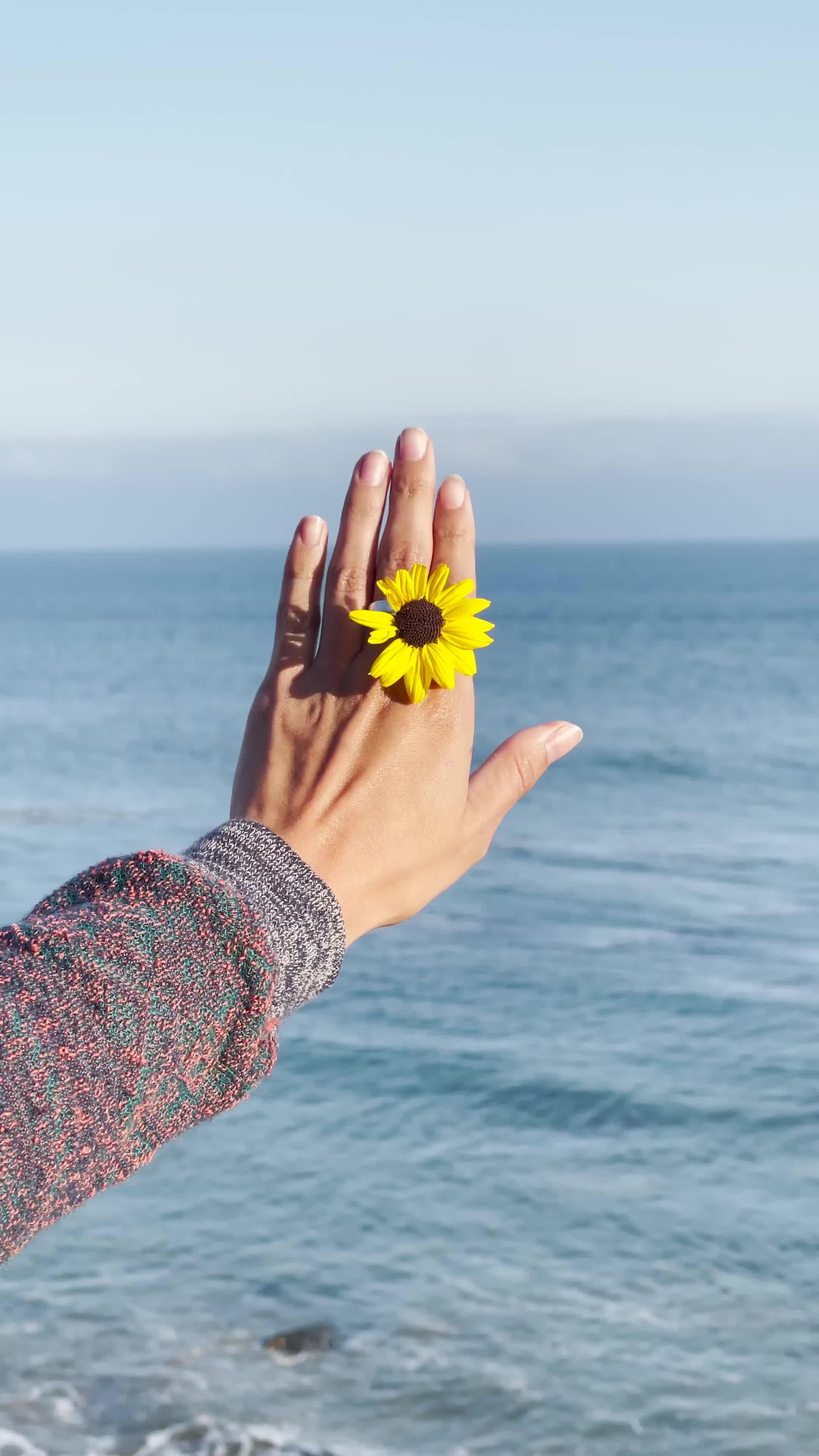
Setting up the React application
Now that we’ve seen how URL transformations for videos work with ImageKit, let’s implement it in a React application.
We will use Create React App to quickly spin up our project:
npx create-react-app video-crop-resize-demo
cd video-crop-resize-demo
Install the ImageKit React SDK:
npm install imagekitio-react
To render the video in your react app, you’ll need your URL endpoint and the file’s path in the media library. You can get your URL-endpoint and ImageKit ID from the developer options section of the dashboard.
In src/App.js
:
import "./App.css";
import { IKVideo } from "imagekitio-react";
function App() {
const urlEndpoint = "https://ik.imagekit.io/<IMAGEKIT-ID>";
return (
<div className="App">
<h1>Resize and Crop videos using ImageKit</h1>
<IKVideo
urlEndpoint={urlEndpoint}
path="/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4"
controls={true}
/>
</div>
);
}
export default App;
The above IKVideo
component renders the following element in the DOM:
<video controls>
<source src="https://ik.imagekit.io/<IMAGEKIT-ID>/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4" type="video/mp4">
</video>
Now we are able to render videos in our react application using ImageKit.
Resizing videos
We can resize the video using the parameters provided in the transformation
prop to IKVideo
.
We can specify the width of the output video using the following code snippet:
<IKVideo
urlEndpoint={urlEndpoint}
path="/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4"
transformation={[
{
width: "300",
},
]}
controls={true}
/>
This renders the following element in the DOM:
<video controls>
<source src="https://ik.imagekit.io/<IMAGEKIT-ID>/tr:w-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4" type="video/mp4">
</video>
Notice the transformation parameter added in the URL:
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:w-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
When only the width is specified for the video, the height is adjusted accordingly to preserve the original video's aspect ratio.
Similarly, height of the video can be specified like this:
<IKVideo
urlEndpoint={urlEndpoint}
path="/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4"
transformation={[
{
height: "300",
},
]}
controls={true}
/>
Which will render the video using the following URL:
https://ik.imagekit.io/<IMAGEKIT-ID>/tr:h-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4
Just like the width transformation, the aspect ratio is preserved in the video for the required height.
We can also control the aspect ratio of the video by specifying the proportions of the required width and height. For example:
<IKVideo
urlEndpoint={urlEndpoint}
path="/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4"
transformation={[
{
ar: "9-16",
width: "300",
},
]}
controls={true}
/>
This generates the following video element:
<video controls>
<source src="https://ik.imagekit.io/<IMAGEKIT-ID>/tr:ar-9-16,w-300/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4" type="video/mp4">
</video>
Cropping videos
We can do the cm-pad_resize
crop on the video using the SDK like this:
<IKVideo
urlEndpoint={urlEndpoint}
path="/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4"
transformation={[
{
height: 450,
width: 800,
cropMode: "pad_resize",
bg: "blurred",
},
]}
controls={true}
/>
This generates the following video element and URL:
<video controls>
<source src="https://ik.imagekit.io/<IMAGEKIT-ID>/tr:h-450,w-800,cm-pad_resize,bg-blurred/pexels-jill-burrow-8953678-uhd_2160_3840_30fps.mp4" type="video/mp4">
</video>
Here’s a table of different crop and crop modes in ImageKit:
Crop / Crop Mode Strategy | Transformation | Behaviour |
---|---|---|
Pad resize crop | cm-pad_resize | Resizes the video to the exact width and height requested, maintains the original aspect ratio, and pads leftover space to avoid cropping or distortion. |
Forced crop | c-force | Forces the video to match the exact width and height requested, ignoring the original aspect ratio. This can cause distortion (stretching or squeezing). |
Max-size crop | c-at_max | Ensures the video fits within the specified width and height while maintaining the aspect ratio. No cropping or distortion; one dimension may scale down. |
Min-size crop | c-at_least | Ensures the video dimensions are equal to or larger than the specified width and height while maintaining the aspect ratio. One dimension will match the requested size, and the other will be scaled up proportionally (no cropping). |
Maintain ratio crop | c-maintain_ratio | This is the default strategy. If no crop is specified, this gets applied. Resizes the video to the requested dimensions while maintaining the aspect ratio and then crops extra parts to achieve the exact width and height. |
Extract crop | cm-extract | Extracts a region of the requested width and height directly from the original video, maintaining the aspect ratio. Unlike c-maintain_ratio, no resizing is performed—only the requested portion is extracted. |
Conclusion
- Setting
height
andwidth
attributes on thevideo
tag only adjusts the display size, and doesn’t actually resize or crop the video file. - Server-side implementations for video transformations solve the problem but involve setting up transformation pipelines, storage, and caching, which is complex to implement.
- ImageKit offers an out-of-the-box solution that handles video storage, transformations (crop, resize, etc.), and optimized delivery with simple URL transformation parameters.
- The React SDK makes video embedding and transformation easy, reducing both development effort and page load times.
- Using the React SDK, you can also apply transformations like text and image overlays on videos and upload files to the media library.