Images are essential to every online business, from e-commerce platforms to content-driven websites. For instance,
- Sellers on marketplaces such as OLX, Amazon or Facebook Marketplace rely heavily on product images to create compelling listings.
- Similarly, user-uploaded review images on sites like Amazon offer potential buyers real-world insights into products.
- Moreover, in the backend operations of these websites, teams frequently manage and upload images, such as product photos, banners, and ads, into their content management systems.
To build these modern media-heavy web applications, you need a powerful storage API solution that is easy to use, secure, fast, scalable, and cost-efficient. You also need a user-friendly interface for non-technical teams in the organization to manage and search those media assets.
👉 Discover different storage API solutions, e.g., popular object storage, disk-based web server storage, and headless DAM. More importantly, learn about the limitations of each approach.
👉 10-line code - Image upload example in Python, React, and many other languages using ImageKit.io storage API.
👉 Finally, learn how to empower non-technical teams in your organization using a powerful media library - uploading, searching, AI-powered background removal, basic resizing, etc.
Different storage API solutions
Let's first understand the different image & video storage solutions available, along with their challenges. We have 3 options:
- Cloud-based object storage
- Disk storage on web servers
- Headless DAM like ImageKit
Cloud-based Object Storage
Cloud providers such as AWS S3, Google Cloud Storage, and Microsoft Azure Blob Storage offer scalable, unlimited object storage solutions with robust APIs for managing digital content. SDKs are available in multiple programming languages for easy integration.
Limitations:
- Although they provide vast storage capabilities, these solutions lack features such as image resizing, optimization, watermarking, and text overlays.
- Additionally, they do not offer robust search or organization features, making it difficult to retrieve or categorize objects beyond basic operations like storing an object at a specific key or listing objects via a prefix. They also lack a user interface for non-technical teams, further limiting their accessibility.
Disk Storage on Web Server
A common method used in platforms like WordPress or Magento, where images are stored directly on the server's disk.
Limitations: This approach has potential scaling and security challenges. The upload API can be an entry point for hackers to inject malicious files, potentially compromising your entire infrastructure.
Headless DAM like ImageKit
This storage solution includes an easy-to-use API and a user-friendly interface for searching and managing media assets. It's designed to streamline the handling of digital content, making it accessible even to those with limited technical skills. Learn more about headless DAM.
Limitations: A hard dependency on third-party tools.
Image Storage API with ImageKit's Media Library
ImageKit is a complete media storage, management, and delivery solution that helps you store, manage, and deliver perfect images across all devices on the web.
ImageKit comes integrated with an image storage solution called the Media Library. The Media Library is built on top of AWS S3, the leading cloud storage provider, and is available in six regions across the globe.
Image Storage APIs and SDKs
ImageKit's Media Library offers unlimited storage and several media management APIs, which allow you to programmatically perform any operation on your images.
ImageKit also provides official SDKs in multiple languages for server-side and client-side operations.
Image upload example in Python
For example, uploading an image from your Python backend using the image upload API is as simple as this ten-line code.
from imagekitio import ImageKit
imagekit = ImageKit(
private_key='your private_key',
public_key='your public_key',
url_endpoint = 'your url_endpoint'
)
imagekit.upload_file(
file= "<url|base_64|binary>",
file_name= "my_file.jpg",
)
Image upload example in React
Similarly, if you want to upload images to ImageKit's image storage directly from the client's device, you could use one of its frontend SDKs to do so. Here is an example of an image upload using the image storage API from a React Application.
<IKContext publicKey="your_public_api_key" authenticationEndpoint="https://www.your-server.com/auth">
<IKUpload
fileName="file-name.jpg"
onError={onError}
onSuccess={onSuccess}
/>
</IKContext>
We have published tutorials on several platforms:
- PHP image and video upload
- Angular file upload
- React file upload
- Many more getting-started guides cover how to use storage API to upload files.
Where are the hosted images stored?
As mentioned earlier, ImageKit's Media Library is built on top of AWS's S3 object storage. When using the image storage API, ImageKit stores the image you upload in AWS S3 in the selected geographical region for your account. It may also additionally backup the image in an alternate geographical location for better data redundancy.
Therefore, by design, you get the same stability and data integrity as that provided by AWS S3, even with ImageKit's media library.
Advantages of using ImageKit's image storage API over cloud storage
Now, you might be wondering why you would want to use ImageKit's image storage API and the Media Library instead of using AWS S3's storage API when essentially, ImageKit is also storing the images in S3.
Let's look at the advantages of ImageKit's storage over traditional cloud storage like S3.
Better image organization and search
In cloud storage like AWS S3, you can store the image on specific keys that give the impression of folder organization. However, with ImageKit's storage API, you can organize images in actual folders.
And for another layer of organization, you can add tags to them. These tags can be either added automatically with AI-based extensions (more on this later in point 3) or can be added manually.
For example, the image storage API below adds the tags 'mobile' and 'halloween sale' to the image 'banner.jpg' and uploads it to the folder 'website_banners'.
imagekit.upload_file(
file= "<url|base_64|binary>",
file_name= "banner.jpg",
options= {
"folder" : "/website_banners/",
"tags": ["mobile", "halloween sale"]
}
)
Now, these tags come in handy when you have to search for specific images, especially when more than one image has the same name.
In the above example, you can use the ImageKit list and search API as shown below to search for Halloween banners for mobile devices using these tags uploaded within the last 7 days and more than 2MB in size.
from imagekitio import ImageKit
imagekit = ImageKit(
private_key='your_private_api_key',
public_key='your_public_api_key',
url_endpoint = 'https://ik.imagekit.io/your_imagekit_id/'
)
list_files = imagekit.list_files({
"tags": ["mobile","halloween sale"],
"searchQuery": 'createdAt >= "7d" AND size > "2mb"'
})
The same robust search functionality is available for non-technical teams through an intuitive user interface (UI) in the Digital Asset Management (DAM) system. For example, search results will display assets that have one or more tags corresponding to the search query, such as "building".
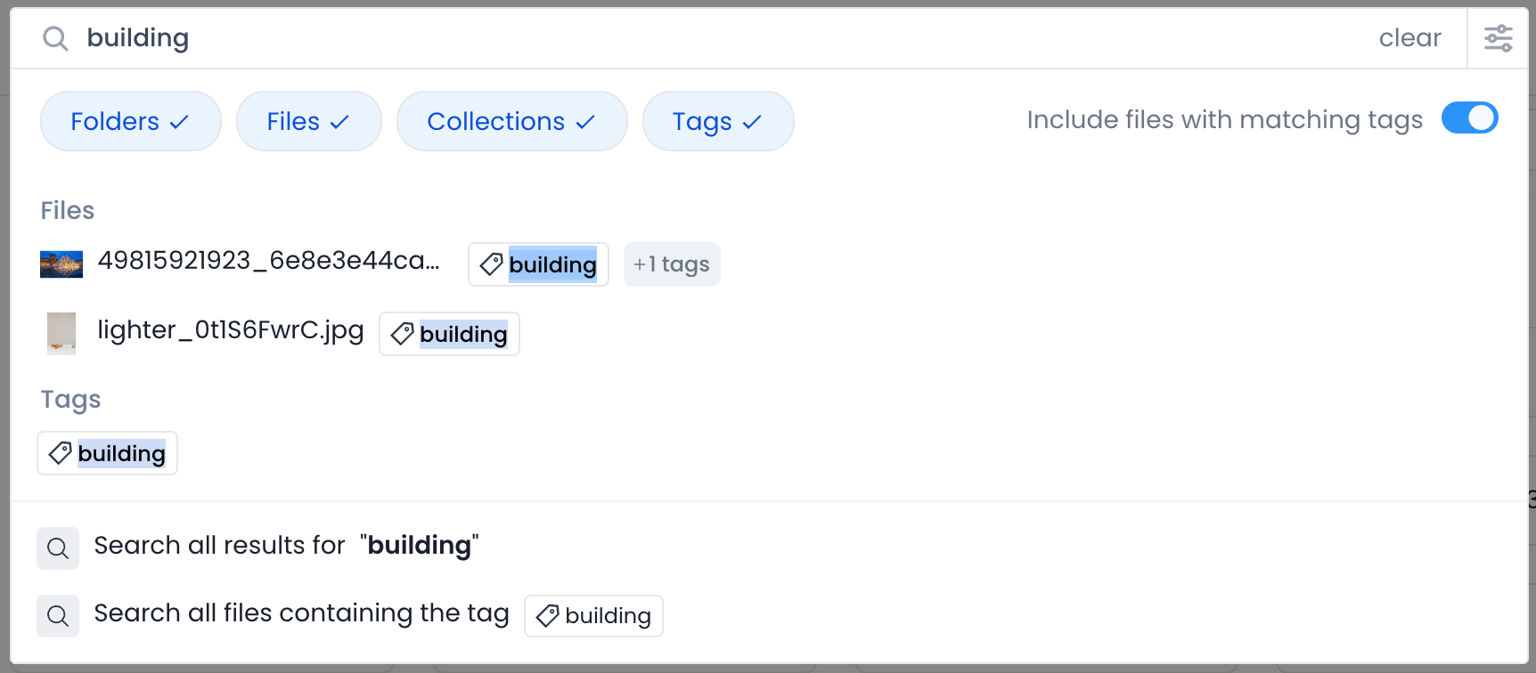
Managing images via API in ImageKit's media library
Once you upload an image using the image storage API, you will have to carry out basic operations such as copying, moving, deleting, or updating these images later.
ImageKit provides a set of image management APIs that allow you to manage the images stored in its storage. These APIs, therefore, allow you to integrate image hosting and management within your existing workflows programmatically.
For example, using ImageKit's NodeJS SDK, you can copy an image from one folder to the other as shown below.
imagekit.copyFile("sourceFilePath", "destinationPath").then(response => {
console.log(response);
}).catch(error => {
console.log(error);
});
Note: These features are also available in ImageKit's dashboard via the UI. You can add multiple users from marketing and content teams and access the ImageKit Media Library to provide an easy-to-use interface to upload and manage the images.
AI-powered image tagging and background removal
ImageKit's storage API not just allows you to host images in its storage, but it also integrates with popular third-party APIs to further simplify your workflow.
For example, you can leverage Google Cloud Vision and AWS Rekognition to tag your images automatically via API.
imagekit.upload_file(
file= "<url|base_64|binary>", # required
file_name= "my_file_name.jpg", # required
options= {
"folder" : "/example-folder/",
"tags": ["a-manual-tag"],
"response_fields": ["is_private_file", "tags"],
"extensions": [{
"name": "aws-auto-tagging",
"minConfidence": 80,
"maxTags": 10
},{
"name": "google-auto-tagging",
"minConfidence": 70,
"maxTags": 10
}]
}
)
The response to the above upload API can look like
// Response body for an update/upload API call
{
/*
...rest of the update/upload response fields
*/
"AITags": [
{
"name": "Shirt",
"confidence": 90.12,
"source": "google-auto-tagging"
},
/* ... more googleVision tags ... */
],
"extensionStatus": {
"google-auto-tagging": "success",
"aws-auto-tagging": "pending"
}
}
Using the search API described in the previous point, you can leverage these AI-powered tags to search for your images hosted with ImageKit.
You can also remove an image's background at the time of image upload with ImageKit's integration with the industry-leading remove.bg
API.
See how the entire background from the car's image below can be removed at the time of upload with ImageKit's image upload API.
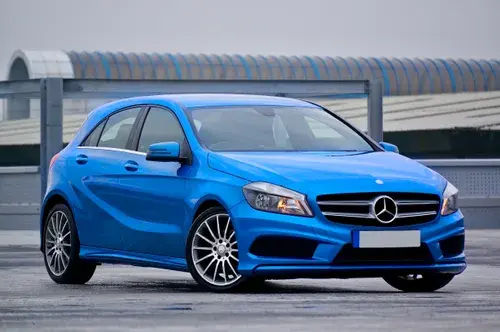

These integrations help you simplify your image hosting and management workflow and make ImageKit's storage a lot more useful than traditional cloud storage like AWS S3 or Google Cloud Storage.
Embeddable in your CMS
The media library widget allows easy integration of ImageKit Media Library into your CMS or any other web application. You can access all the assets stored in your Media Library from your existing CMS or application.
Learn more from docs.
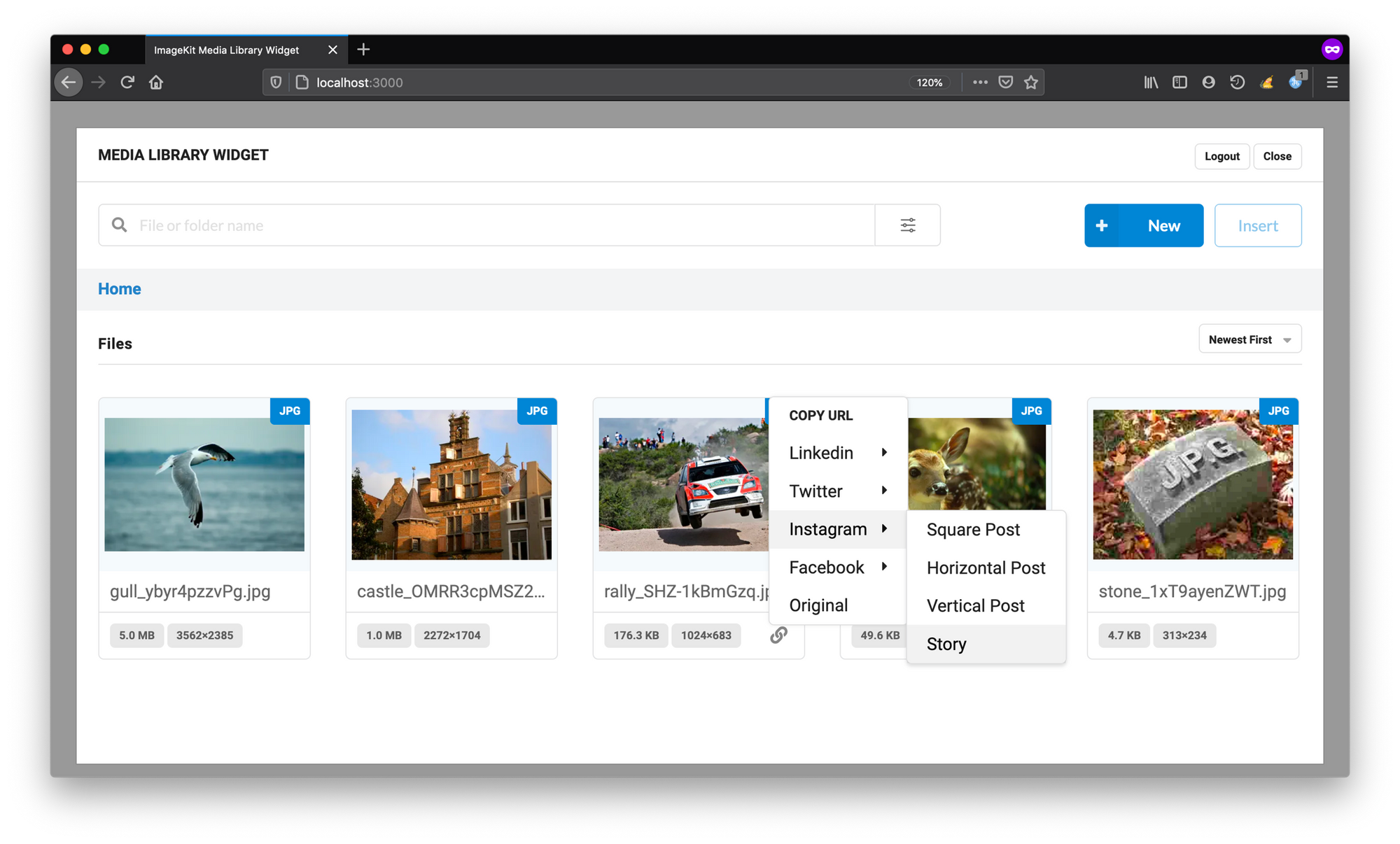
Backing up your images hosted with ImageKit
Images (or content in general) are a critical resource for any business. You spend a lot of time and effort to collate them, and they represent your brand. You would still want a copy of your images outside of ImageKit, for use on other platforms or just to keep them in alternative storage as well.
Unlike other image hosting platforms that lock you in, ImageKit allows you to backup the images hosted in the media library to an AWS S3 bucket of your choice.
Anything that you upload in ImageKit, it also copied to your storage, ensuring you always have a copy of it outside of ImageKit, while still getting the benefits of ImageKit's advanced asset management features while using the media library.
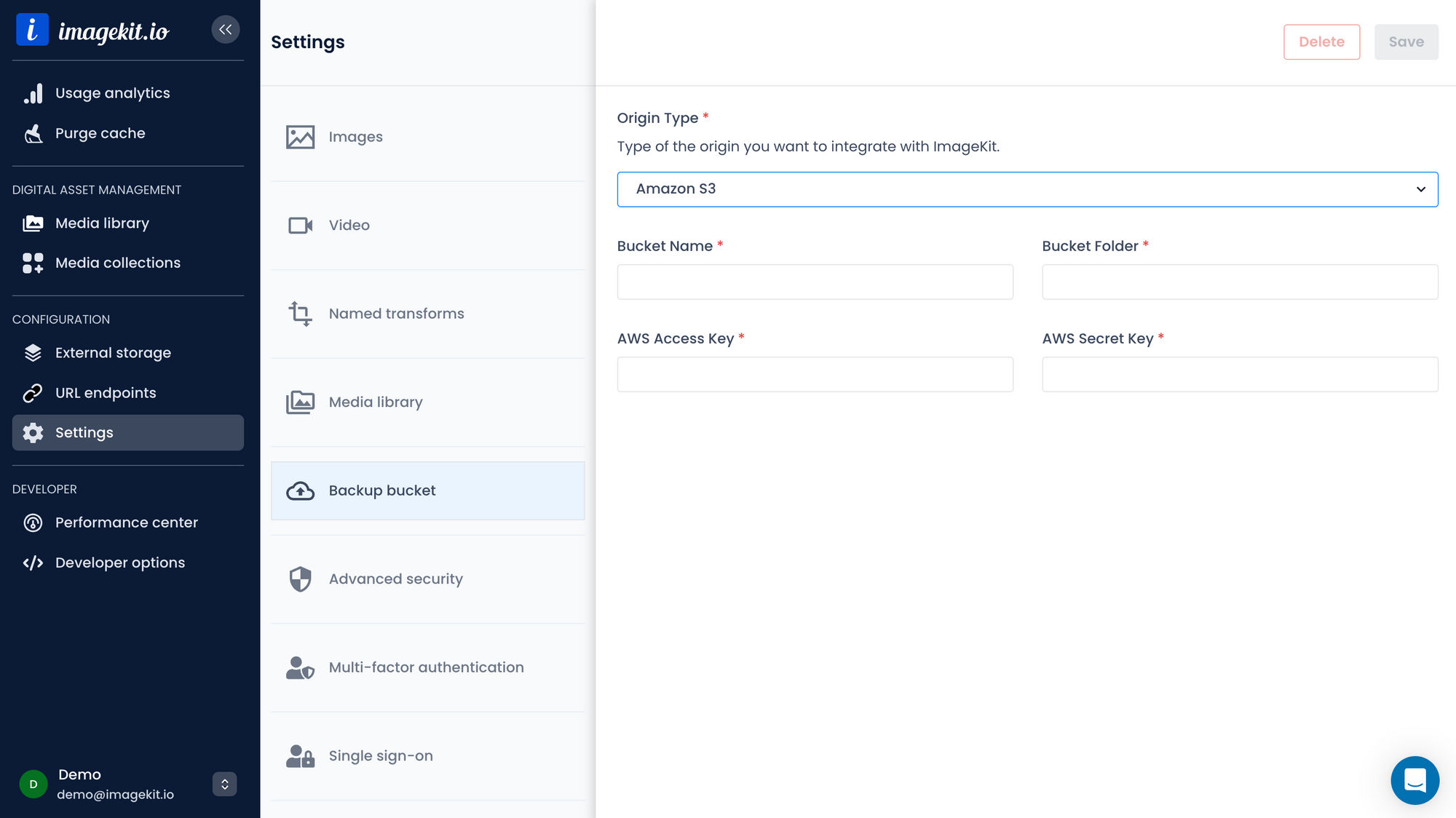
And the best part - Real-time image optimization and transformations
With several different devices and different kinds of content in the modern world, it is essential to have real-time image optimization and transformations to deliver the right experience across all devices.
Cloud storages like S3, Google Cloud Storage, etc., offer image storage solutions but do not come with image optimization or resizing capabilities. Therefore, if you are storing high-quality original images in these storages, you have to either build a solution of your own or use a third-party service to optimize these images for your requirement.
While ImageKit supports image optimization with all popular third-party cloud storage as well, if you use the Media Library to host your images, you get all these capabilities natively with the storage.
Any image hosted in ImageKit's Media Library gets you an image URL. If you use this URL on your website or app, ImageKit will automatically convert the image to the best possible format and compress it in real-time.
For example, the following image is automatically delivered in WebP format on supported devices and compressed.
https://ik.imagekit.io/ikmedia/default-image.jpg
You can also resize an image to any size in real-time and control how it is cropped or how the aspect ratio is preserved. The image URL below has the parameter tr=w-300,h-200
at the end that will get us a 300 x 200px image in real-time.
https://ik.imagekit.io/ikmedia/default-image.jpg?tr=w-300,h-200
ImageKit supports over 40 different real-time image transformations. We could do something more complex like converting this image into a banner with a watermark and dynamic text written on the image in real-time, as shown in the example below. Check out the image URL here.
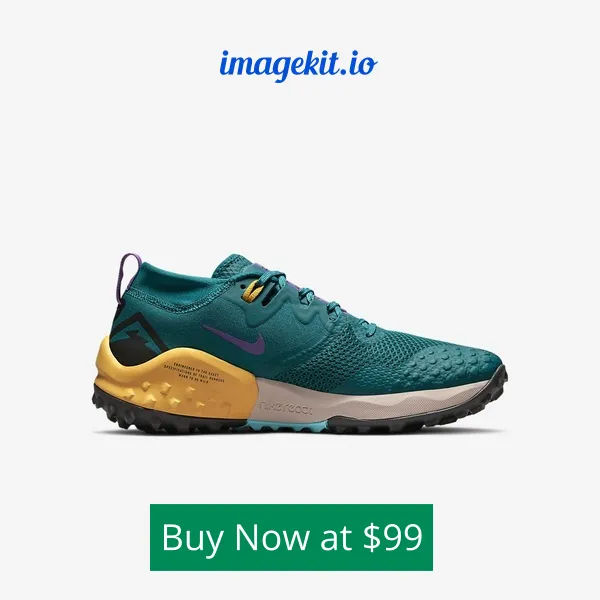
Once the transformation is done in real-time, ImageKit uses AWS CloudFront CDN, which has over 220 server nodes globally, to deliver the image to your users for a faster load time.
Summing it up
The image storage API provided by ImageKit gives you access to a world-class, reliable image hosting service. You can use the storage APIs to upload and manage images and programmatically integrate them with your existing workflows.
With image tagging, AI-powered extensions, advanced search functionality, and a backup to S3 feature, ImageKit's image storage solution is much more powerful than any other standard cloud storage while providing the same level of reliability and uptime.
And with the built-in real-time optimizations and transformations, you won't have to look at any other tool to deliver perfect visual experiences to your users.
So, what are you waiting for? Try out the image storage API by signing up for the Forever Free plan, which provides 5GB of image storage.