Microsoft Azure, often called just Azure, is the cloud service offered by Microsoft that competes with other leading cloud providers like AWS and Google Cloud. It provides access to data centers and services managed by Microsoft.
Similar to the popular AWS S3 storage, Azure too provides an object storage called the Azure Blob Storage. Users can upload different kinds of files like images, videos, documents, zip files, and more without worrying about the storage space available, which is practically infinite.
In this blog, we will look at how we can stream videos with Azure Blob Storage and how we can improve upon it and stream optimized videos using ImageKit's Video API.
Using Azure Blob Storage for video hosting and streaming
For this demonstration, we have uploaded a 14.7MB sample MP4 video test-video.mp4
. We have uploaded this to a container test-public
in our Azure blob storage.

We can generate a Shared Access Signature (SAS) URL in Azure to provide access to this video file directly from the blob storage. SAS makes the URL publicly accessible, and you can also enforce other access restrictions on it. The URL can then be used directly on your website or app to load the video
<video>
<source src="https://bucketname.blob.core.windows.net/test-public/test-video.mp4?sp=r&sv=2021-06-08&sr=b&sig=<signature>......" />
</video>
When you load this video URL on an HTML page, the browser issues multiple range requests to access it in smaller chunks. Notice in the screenshot below that the response status code for the request is 206, indicating a partial response from the server that matches the browser's requested segment.
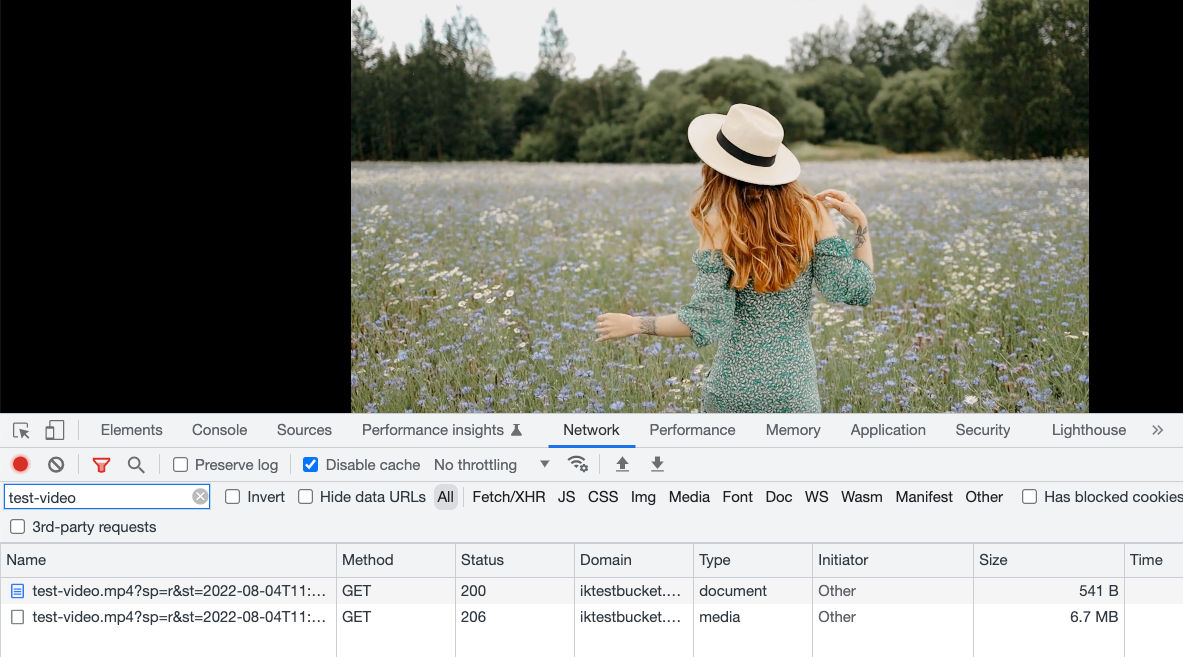
Do note that making an object accessible publicly, as we did above, is generally not recommended.
Microsoft Azure also provides a content delivery network (CDN) as a part of its service. We can connect our cloud storage and deliver our video using the CDN. This change helps keep our blob storage private and speeds up the load time because of the Azure CDN.
While in the above example, the browser was able to request videos in smaller chunks, it is not the "streaming" like Youtube and Netflix. These websites use Adaptive Bitrate Streaming, where a bigger video file is broken into smaller chunks and encoded at different bitrates. The end result is a manifest file, something like a playlist with the names of the different chunks. This manifest file is then provided to a video player, which decides which chunk to play and at what resolution, depending on the user's device and network conditions. You would have noticed that when you access a video on YouTube, the video quality sometimes adjusts to your network speed to ensure continuous playback.
Adaptive Bitrate Streaming with Microsoft Azure's Media Services - the difficult way
To stream your videos using DASH or HLS Adaptive Bitrate Streaming protocols or to perform any other optimization, Azure provides a suite of transcoding services under its product called Media Services. You can store your original video in the Blob storage and then use the Media Services to encode the video into a suitable streaming format.
This, however, requires a deep understanding of the different parts of the Media Services offering, about video and audio encoding and formats, and how to best use them. The configurations and setup of media services, a part of which is provided here, are complex and have a steep learning curve.
While the flexibility and the features offered by Azure Media Services are great, it isn't easy to start. When you want to deliver video experiences on your website and app, you want to use a tool that can make it dead simple to achieve standard optimizations and transformations without extensive documentation. This is where ImageKit comes in with its super simple, URL-based real-time video API.
Reprocessing videos to a new variant with Media Services
If you have a vast video repository and decide to make a change in the future, let's say you want to change your watermark on them, you will have to re-process all the videos. That would mean creating a new config, running it on all the existing videos, and ensuring it gets completed. That's a lot of development effort and additional spending on cloud storage.
Simplifying video streaming and optimizations with ImageKit's video API
Instead of spending hours learning the intricacies of Azure and media streaming and encoding, you could spend a few minutes configuring your Azure Blob Storage with ImageKit's real-time video API and start streaming optimized video content to your user's device.
ImageKit is a real-time media optimization, transformation, and management solution that allows us to stream and optimize videos in near real-time with minimum effort. It uses an integrated global CDN for media delivery, so you don't have to worry about content delivery times for your users either.
And the best part is that it natively integrates with popular cloud storages, including Azure Blob Storage, whether private or public. You can therefore start using it to stream optimized video content with minimal effort.
You can sign up for a free ImageKit account from here. It offers free 500 seconds of video processing and 20GB of data output every month.
Let's use the 14.7MB test-video.mp4
that we loaded to the blob storage above and see how we can optimize it with ImageKit.
Connecting our Azure Blob storage to ImageKit
Besides providing built-in media storage, ImageKit can connect to popular cloud storage, including Azure Blob Storage.
Read more on configuring external storage with ImageKit
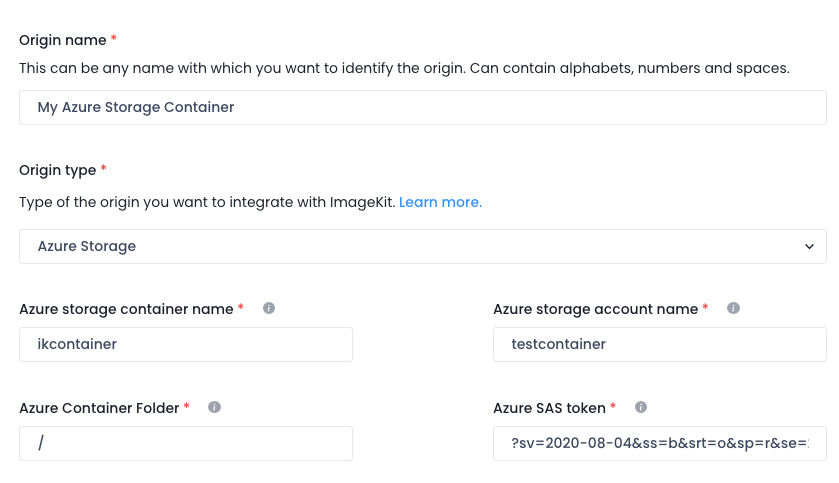
We have to then attach this external storage to a URL endpoint in our dashboard, making it accessible via ImageKit. ImageKit does not copy any content from your blob storage. Instead, the above integration provides access to the original file when you request it via a URL.
With our storage now attached to ImageKit, let's see how we can optimize the original video for delivery on our user's device.
Streaming optimized video with ImageKit and Azure Blob Storage
Using the URL below, we can access our video stored in the cloud using ImageKit.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4
When you load a video using this URL, ImageKit automatically identifies the requesting device and its video format support to convert the original video to WebM or MP4, the two most widely used web-safe video formats. ImageKit also compresses the video in real-time without significantly degrading its visual quality.
Unlike other third-party tools, which involve extensive setup or understanding of the video encoding process, these video optimizations are accessible in the ImageKit dashboard. You need to turn on the settings, and ImageKit takes care of the rest when you deliver the video using its URL.
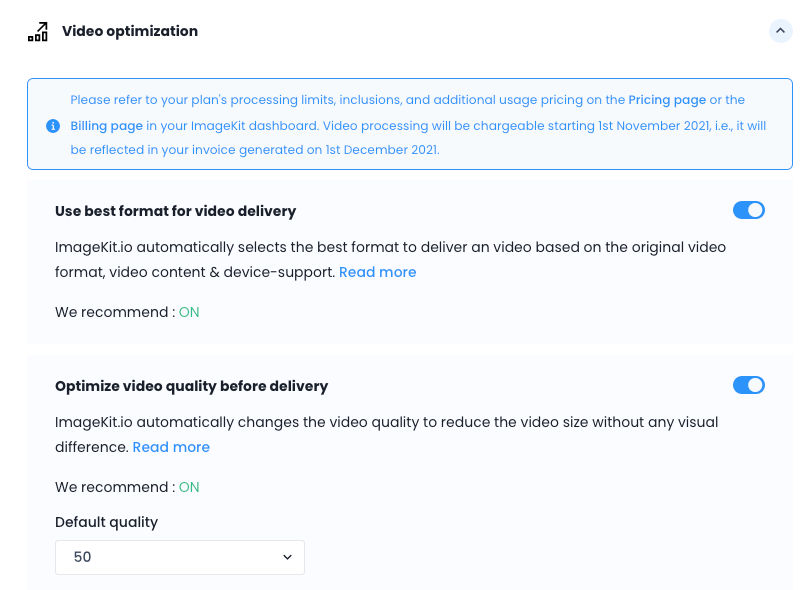
When we deliver this video via ImageKit, its goes from 14.7MB to just 9.1MB when compressed and delivered in WebM format on Chrome.
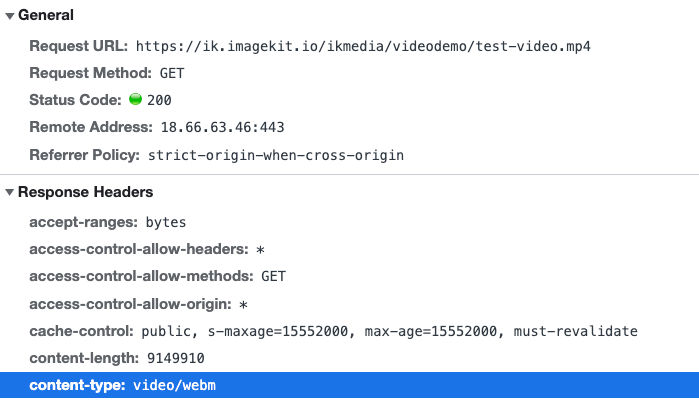
We didn't have to set up anything, learn about video encoding, or write any code. Delivering the file via ImageKit is enough to get a 40% lighter video streaming experience.
Transforming videos for different use cases with ImageKit
ImageKit offers a URL-based real-time video API, making it easy to transform videos to adapt them to different devices and placeholders. You can resize and crop videos, watermark them, create video snippets, or create thumbnails in real-time. These transformations help you deliver a complete video experience on the client's device without any other external product or coding.
To scale our video to a 200px width, we have to add the video transformation parameter tr=w-200
to our URL, as shown below.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-200
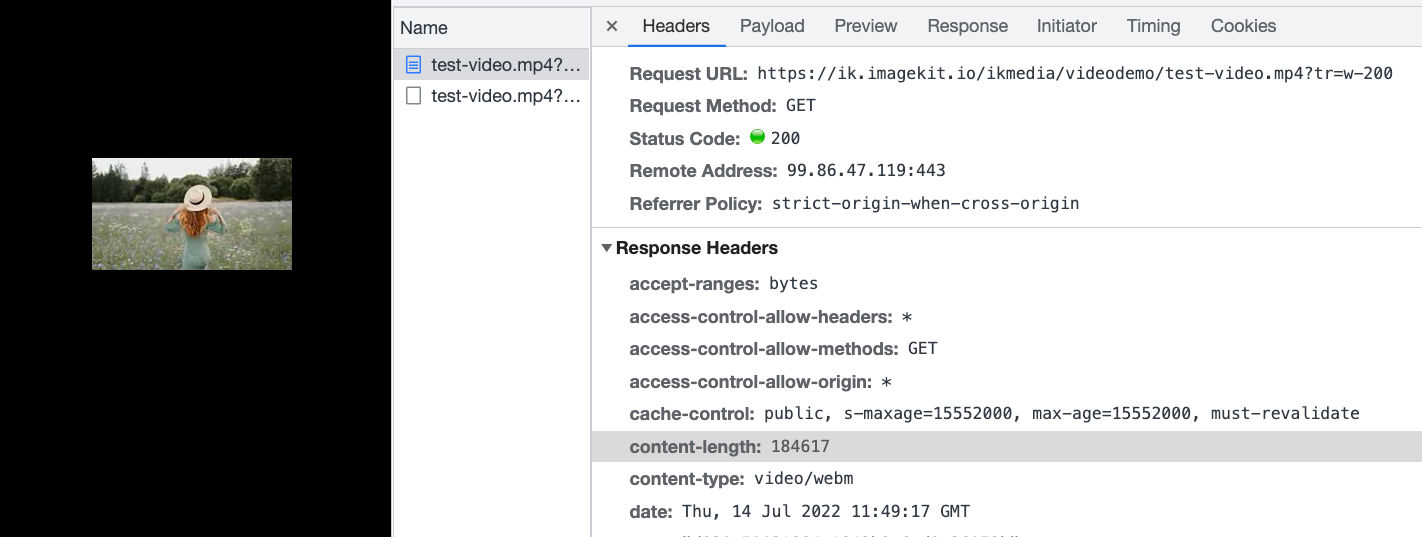
While resizing the video, ImageKit still performs the format and quality optimization we saw earlier. The video gets compressed to 184KB and is again delivered in WebM format.
What if we want to tailor our video to Instagram reels or any other platform that requires a vertical video?
In that case, we can do so by specifying the appropriate height and width transformations directly in the video URL. ImageKit takes care of cropping the video in real-time and returns the size we requested. For a 400 x 640 vertical video requested using the URL below, we get the video size at 2.3MB.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-400,h-640
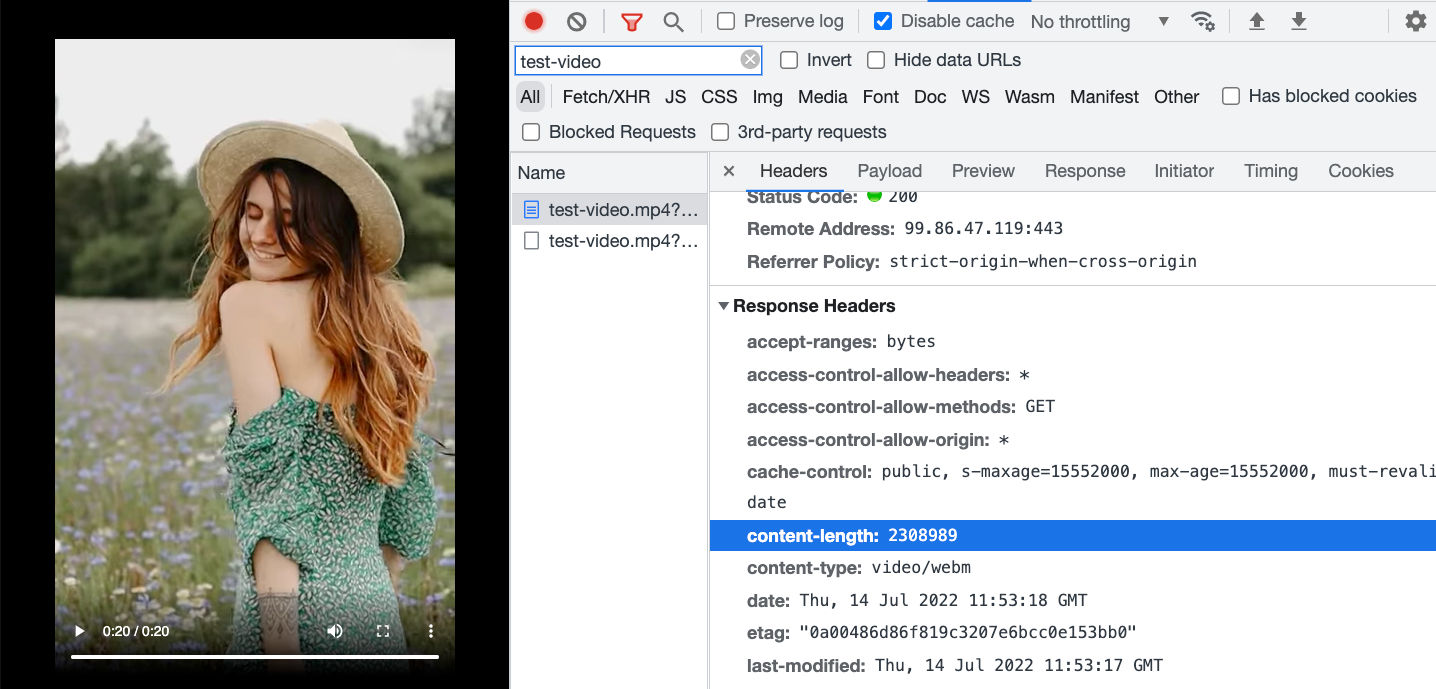
We can also watermark our video and control the placement and other formatting options of the watermark in real-time. This helps us in adding our branding to the video in real-time. The example below uses the concept of layers in ImageKit's transformation to place an image on the underlying video. You could also add text or another video on top of a base video. Again, no extensive setup. Just a URL change does it.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-400,h-640,l-image,i-logo_HuFO6vJ2x.png,lx-10,ly-10,w-200,l-end
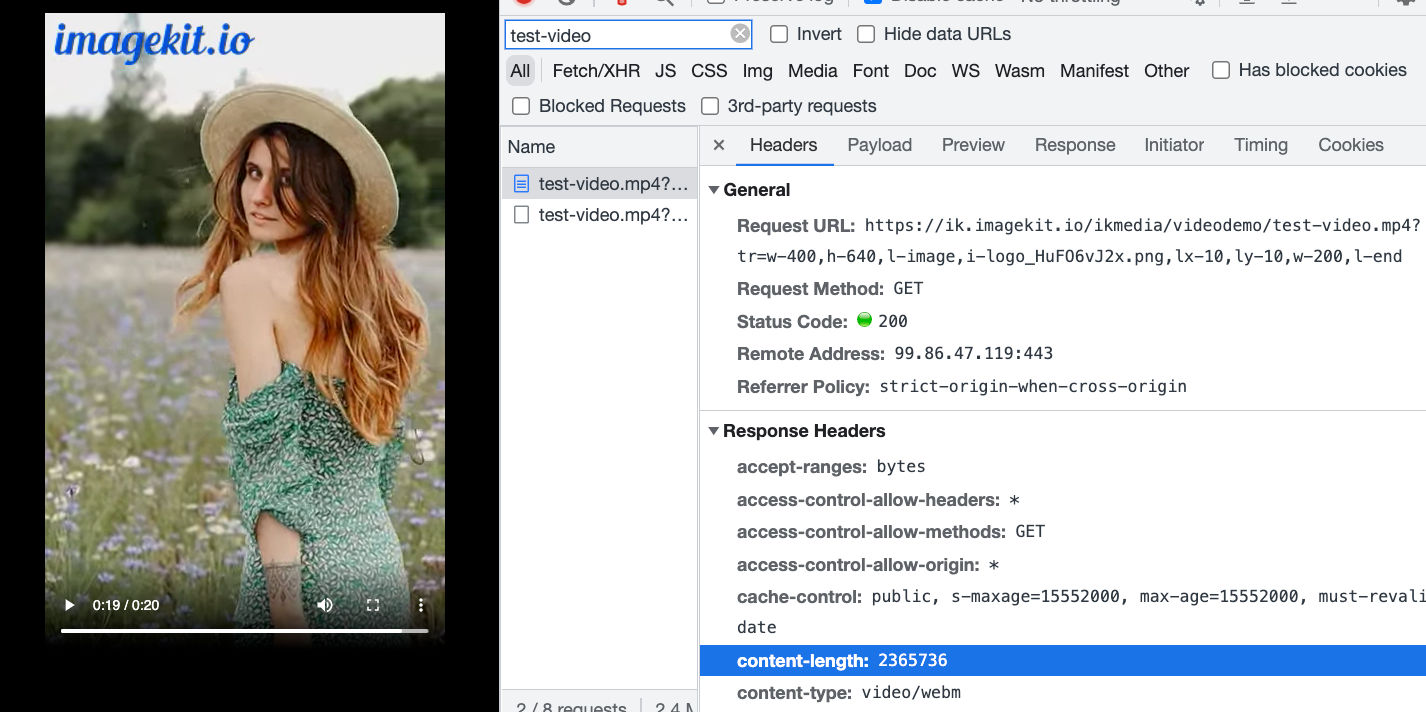
All the optimizations and transformations we saw above do not require using any particular video player like video.js. You can just load the URLs along with their transformation parameters directly in a video tag on your website, and the browser will be able to load them.
Comparing the re-processing of videos with Azure Blob Storage
When using the Media services offered by Azure, making the smallest changes would require re-processing all our videos. With ImageKit, you don't have to re-run the processing jobs. Make the change you want in the URL, which will automatically apply to all videos when a request comes in. If a video is no longer requested, it will not get processed.
Adaptive bitrate streaming for videos with Azure Blob Storage and ImageKit
Adaptive bitrate streaming is a technique that involves encoding videos at different bitrates and resolutions and loading them as per the user's network speed or device changes. All popular streaming services extensively use this, and most of us would have experienced this adjustment in quality ourselves.
ImageKit makes it super simple to use DASH and HLS - the two most popular Adaptive Bitrate Streaming protocols with a URL-based API. Like all other video optimizations and transformations in ImageKit, Adaptive Bitrate Streaming can also be used in seconds, and in real-time, by making small URL changes.
For example, we can get an HLS stream ready at 360p, 480p, and 720p resolutions by modifying our video URL, as shown below.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4/ik-master.m3u8?tr=sr-360_480_720
Compare this URL to the code we had earlier for a similar job with Azure's Media Services. The above URL change is far simpler.
When accessed for the first time, ImageKit will automatically start transcoding our video into different resolutions in the background and return the manifest file - ik-master.m3u8
- for HLS streaming. It then stores the manifest file and the various chunks internally and keeps them ready for delivery when a video player requests them.
We can load the above URL directly in an HLS video stream player. That's it! Our adaptive bitrate streaming setup is ready.
We can see in the screenshot below that the browser first started with a couple of 360p video segments (360p-segs_00000.ts
) for a fast load time before switching up the resolution to 720p because the user's device could load a higher resolution video.
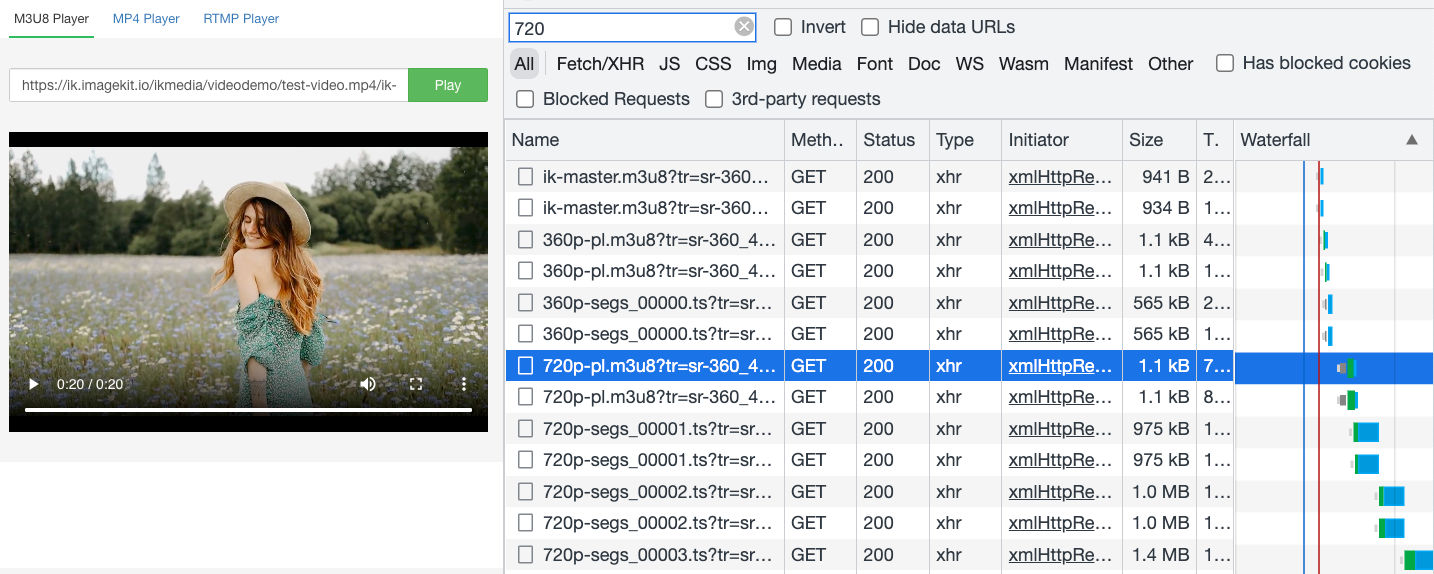
Since video files are large, generating manifest files and the corresponding chunks at multiple resolutions for Adaptive Bitrate Streaming is time-consuming. To improve the development experience and provide certainty about when the asset is ready, ImageKit offers video webhooks. These webhooks allow us to listen to events indicating the completion of this transcoding process. These webhooks are optional, and the process of generating ABS manifests, as explained above, remains the same whether or not we use them.
{
"type": "video.transformation.ready",
"id": "a03031b5-ad5f-4985-8cf5-4de67630f6d7",
"created_at": "2022-06-20T12:00:11.703Z",
"request": {
"x_request_id": "fa98fa2e-d6cd-45b4-acf5-bc1d2bbb8ba9",
"url": "http://ik.imagekit.io/demo/sample-video.mp4?tr=f-webm,q-10",
"user_agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:101.0) Gecko/20100101 Firefox/101.0"
}
....
other fields
....
}
Conclusion
Azure Blob Storage is the object-storage solution provided by Microsoft Azure Cloud. It is very similar to AWS's S3 object storage. We can upload video files to it and load them on the user's device.
We can also use the Media Services product in Azure to get ABS for our videos, crop them or watermark them. However, setting up Media Services, even for the simplest of transformations, has a steep learning curve, requires extensive setup, and involves significant effort and costs to re-process a video should our delivery requirements change.
ImageKit is a real-time media optimization, transformation, and management product that simplifies streaming optimized videos directly from your Blob storage. Once the storage is attached, we can use the URL-based real-time video API to optimize videos automatically, convert them to the right format, adapt them to web and mobile, and build adaptive bitrate streaming using DASH and HLS protocols.
ImageKit offers a great free plan with almost 500 seconds of SD video processing and 20GB of video streaming every month. Sign up now and use ImageKit with Azure Blob Storage for video streaming and optimization on websites and apps.