Google Cloud is a leading full-service cloud provider that provides access to cloud services that run on the same infrastructure used by Google's public services like Google Search, Gmail, and more.
One of the cloud services provided as a part of Google Cloud is the object storage called Google Cloud Storage. Similar to the S3 offering by AWS, Google cloud storage provides a virtually infinite amount of space to host all your content, including video files.
Using Google Cloud Storage for video hosting and streaming
Google Cloud Storage comes with a dashboard and several APIs that allow you to automate every possible action you intend to take. We can upload video files using the PUT Object API or the Cloud Storage dashboard. For this blog, we have uploaded a 14.7MB sample MP4 video test-video.mp4.
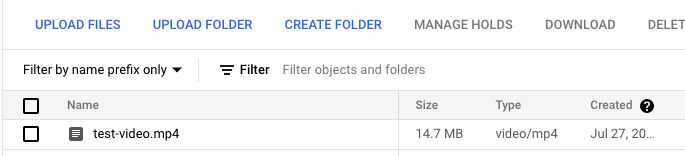
While Google Cloud Storage is primarily an object storage, you can make the object stored in it publicly accessible and use its URL to load your video on any website or app. For example, we can use the video URL in a video tag, as shown below.
<video>
<source src="https://storage.googleapis.com/bucketname/test-video.mp4" />
</video>
When you load this on an HTML page, the browser issues multiple range requests to access the video in smaller chunks. These requests are completed with a 206 status code indicating a partial response from the server that matches the browser's requested segment.
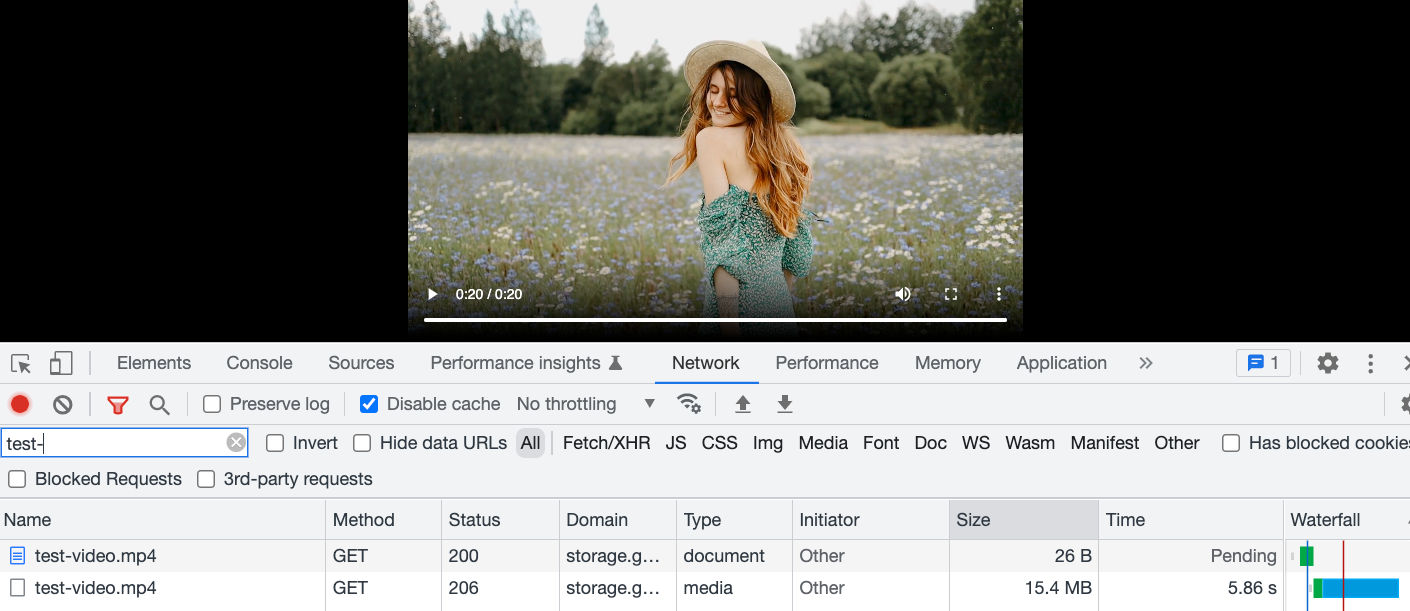
Making an object accessible publicly as we did above is generally not recommended. Since Google also provides a content delivery network (CDN) as a part of its service, we can connect our cloud storage to it and then deliver our content using the CDN. This change helps keep our storage private and speeds up the load time because of the Google Cloud CDN.
Though we can deliver video content, as shown above, it is not the "streaming" like Youtube and Netflix. These websites use Adaptive Bitrate Streaming, where a bigger video file is broken into smaller chunks and encoded at different bitrates. The bitrate used then depends on the user's device and network conditions. You would have noticed that when you access a video on these websites, it starts playing almost immediately, and the video quality sometimes adjusts to your network speed to ensure continuous playback.
Adaptive Bitrate Streaming with Google Cloud Storage - the difficult way
To support adaptive bitrate streaming and other optimizations with the videos uploaded to your Google Cloud Storage, Google provides another product called the Transcoder API.
For this API, you need to have an input video in one Google Cloud Bucket, provide an output bucket, set up a job with the proper configuration, initiate that job, and then wait for job completion. While this process works, it is time-consuming and requires an extensive setup on the cloud. It also requires in-depth knowledge about API options, video encoding best practices, and how to use the two to achieve different transformations and optimizations.
For example, a job is to generate HLS and DASH manifests for Adaptive Bitrate Streaming at 360p and 720p resolutions.
{
"config": {
"inputs": [
{
"key": "input0",
"uri": "gs://STORAGE_BUCKET_NAME/STORAGE_INPUT_VIDEO"
}
],
"elementaryStreams": [
{
"videoStream": {
"h264": {
"heightPixels": 360,
"widthPixels": 640,
"bitrateBps": 500000,
"frameRate": 30
}
},
"key": "video-stream0"
},
{
"videoStream": {
"h264": {
"heightPixels": 720,
"widthPixels": 1280,
"bitrateBps": 3200000,
"frameRate": 30
}
},
"key": "video-stream1"
},
{
"audioStream": {
"codec": "aac",
"bitrateBps": 64000,
"sampleRateHertz": 48000
},
"key": "audio-stream0"
}
],
"muxStreams": [
{
"key": "video-only-sd",
"container": "fmp4",
"elementaryStreams": [
"video-stream0"
]
},
{
"key": "video-only-hd",
"container": "fmp4",
"elementaryStreams": [
"video-stream1"
]
},
{
"key": "audio-only",
"container": "fmp4",
"elementaryStreams": [
"audio-stream0"
]
}
],
"manifests": [
{
"fileName": "manifest.m3u8",
"type": "HLS",
"muxStreams": [
"video-only-sd",
"video-only-hd",
"audio-only"
]
},
{
"fileName": "manifest.mpd",
"type": "DASH",
"muxStreams": [
"video-only-sd",
"video-only-hd",
"audio-only"
]
}
],
"output": {
"uri": "gs://STORAGE_BUCKET_NAME/STORAGE_OUTPUT_FOLDER"
}
}
}
While the options above provide a lot of flexibility, getting started with this API has a steep learning curve. We will simplify this A LOT with ImageKit's URL-based video API.
Also, if you have a vast video repository and decide to make a change in the future, let's say you want to change your watermark on them, you will have to re-process all the videos. That's a lot of development effort and additional spending on cloud storage.
Simplifying video streaming and optimizations with ImageKit's video API
Alternatively, you could spend a few minutes configuring your Google Cloud storage with ImageKit's real-time video API and start streaming optimized video content to your user's device.
ImageKit is a real-time media optimization, transformation, and management solution that allows us to stream and optimize videos in near real-time with minimum effort. It uses an integrated global CDN for media delivery, so you don't have to worry about content delivery times for your users either.
And the best part is that it natively integrates with Google Cloud Storage, whether private or public, and you can therefore start using it to stream optimized video content with minimal effort.
You can sign up for a free ImageKit account from here. It offers free 500 seconds of video processing and 20GB of data output every month.
Let's use the 14.7MB test-video.mp4
that we loaded to Google Cloud above and see how we can optimize it with ImageKit.
Connecting our cloud storage to ImageKit
Besides providing built-in media storage, ImageKit can connect to popular cloud storage, including Google Cloud Storage.
Read more on configuring external storage with ImageKit
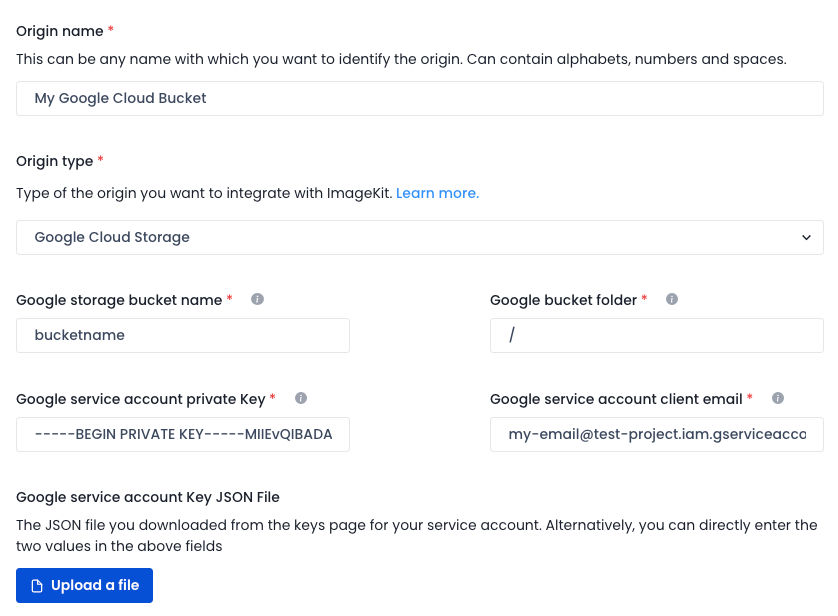
We must then attach this external storage to a URL endpoint in our dashboard, making it accessible via ImageKit. ImageKit does not copy any content from your storage. Instead, it just accesses the original file when you request it via a URL.
With our storage now attached to ImageKit, let's see how we can optimize the original video for delivery on our user's device.
Streaming optimized video with ImageKit and Google Cloud Storage
Using the URL below, we can access our video stored in the cloud using ImageKit.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4
When requested using this URL, ImageKit automatically identifies the requesting device and its video format support to convert the original video to WebM or MP4, the two most widely used web-safe video formats.
Using video compression algorithms, ImageKit also compresses the video without significantly degrading its visual quality.
Unlike other third-party tools, which involve extensive setup or understanding of the video encoding process, these video optimizations are accessible in the ImageKit dashboard. You need to turn on the settings, and ImageKit takes care of the rest when you deliver the video using its URL.
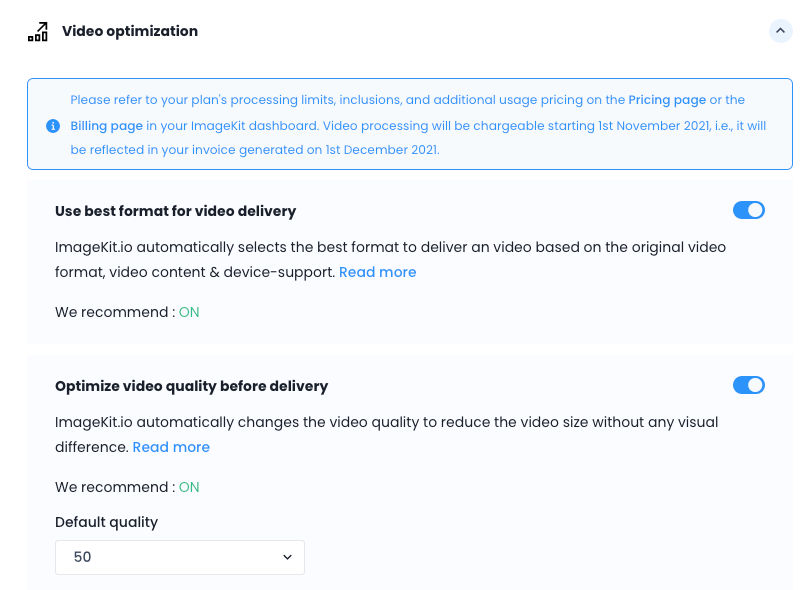
When we deliver our sample video via ImageKit, its size drops from 14.7MB to just 9.1MB when delivered in WebM format on Chrome.
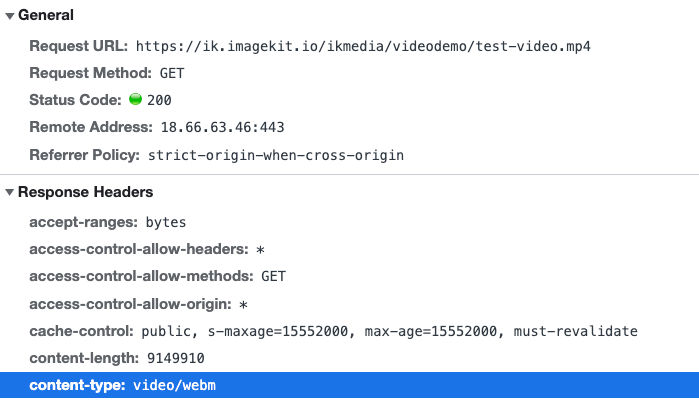
We didn't have to set up anything or learn about video encoding. Delivering the file via ImageKit is enough to get a 40% lighter video streaming experience.
Transforming videos for different use cases with ImageKit
ImageKit offers a URL-based real-time video API, making it easy to adapt videos to different devices and placeholders. You can resize and crop videos, watermark them, create video snippets, or create thumbnails in real-time. These transformations help you create a complete video experience on the client's device without any other external product or coding.
To scale our video to a 200px width, we have to add the video transformation parameter tr=w-200
to our URL, as shown below.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-200
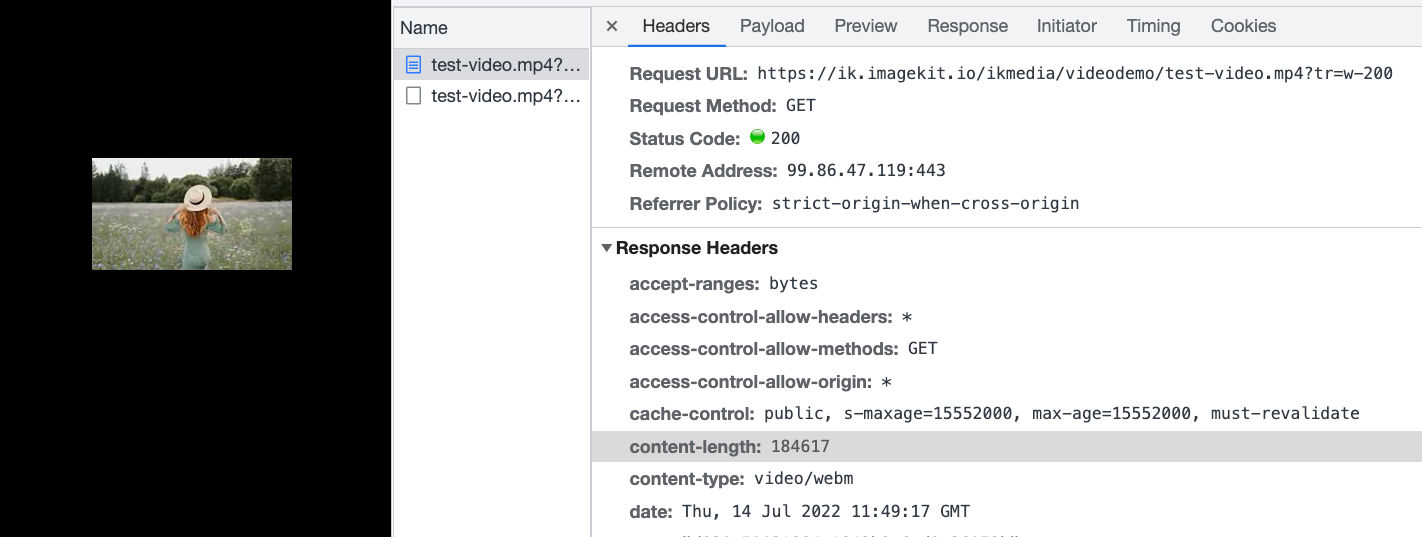
While resizing the video, ImageKit also converts it to WebM format because we access it from a browser that supports it. The video gets compressed to just 184KB.
Suppose we want to tailor our video to Instagram reels or any other platform that requires a vertical video. In that case, we can do so by specifying the appropriate height and width transformations directly in the video URL. ImageKit takes care of cropping the video in real-time and returns the size we requested. For a 400 x 640 vertical video requested using the URL below, we get the video size at 2.3MB.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-400,h-640
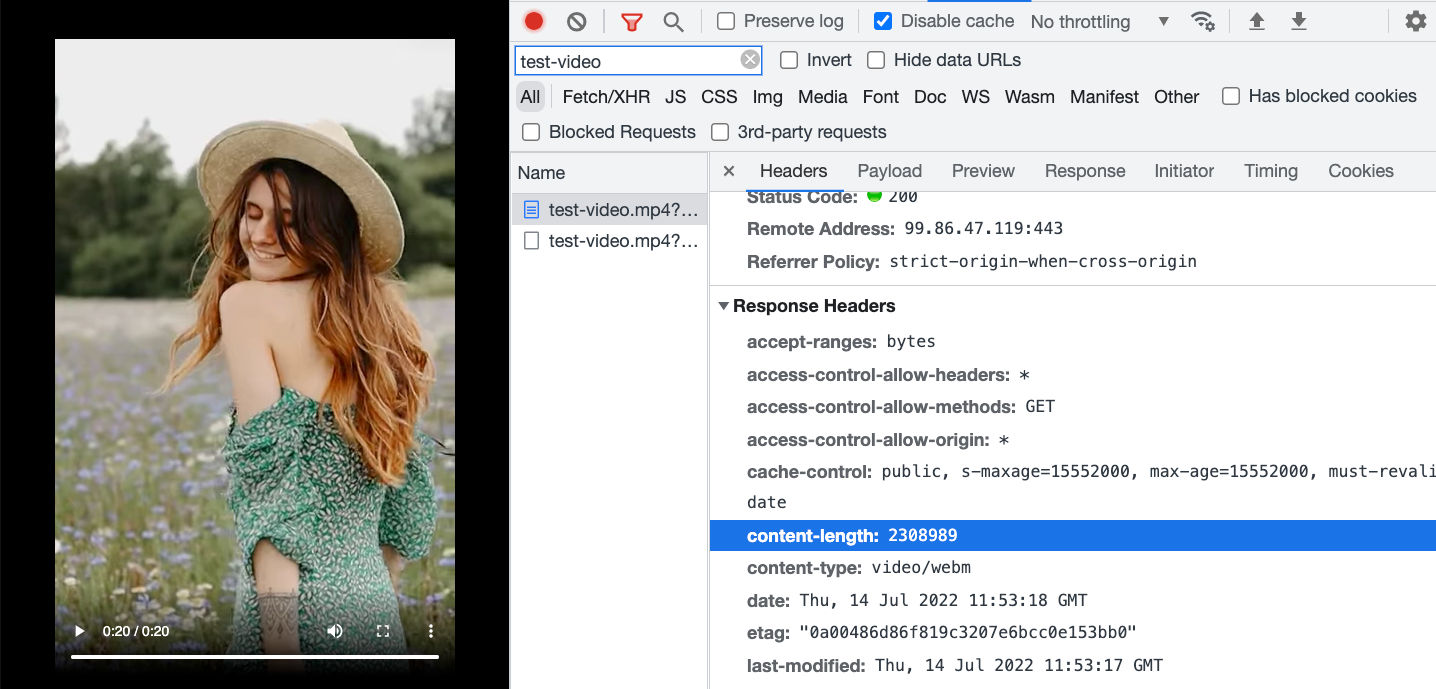
Taking it a step further, we can watermark our video and control its placement and other formatting options in real-time. The example below is an image placed on the underlying video, but you could also add text or another video on top of a base video. Again, no extensive setup. Just a URL change does it.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4?tr=w-400,h-640,l-image,i-logo_HuFO6vJ2x.png,lx-10,ly-10,w-200,l-end
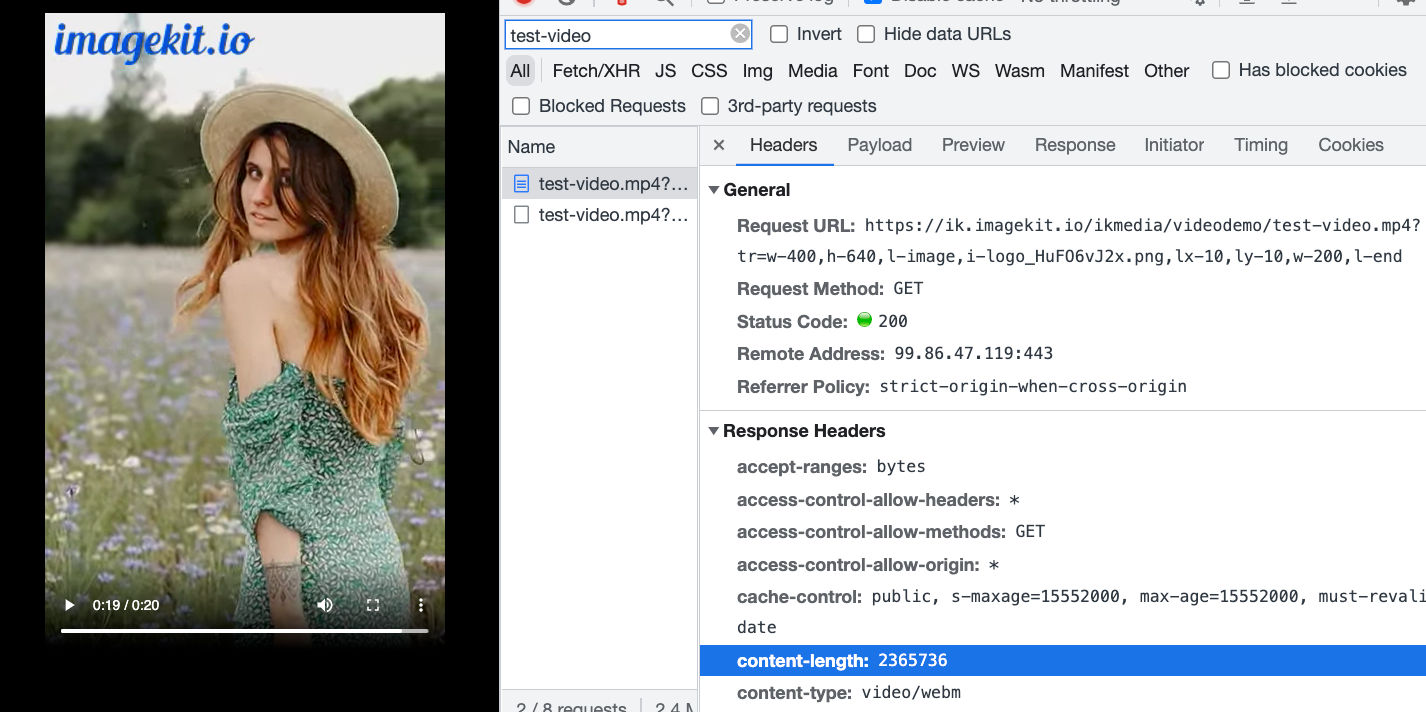
Comparing the re-processing of videos with Google Cloud Storage
When using the Transcoder API, making the smallest changes would require re-processing all our videos. With ImageKit, you don't have to re-run the processing jobs. Make the change you want in the URL, and it will automatically apply to all videos when a request comes in. If a video is no longer requested, it will not get processed.
Adaptive bitrate streaming for videos with Google Cloud Storage and ImageKit
Adaptive bitrate streaming is a technique that involves encoding videos at different bitrates and resolutions and using them according to the user's network speed or device changes. Streaming services such as Netflix and Youtube extensively use this, and most of us would have experienced this adjustment in quality ourselves.
ImageKit makes it super simple to use DASH and HLS - the two most popular Adaptive Bitrate Streaming protocols. Like all other video optimizations and transformations in ImageKit, Adaptive Bitrate Streaming can also be used in seconds, and in real-time, by making small URL changes.
For example, we can get an HLS stream ready at 360p, 480p, and 720p resolutions by modifying our video URL, as shown below.
https://ik.imagekit.io/ikmedia/videodemo/test-video.mp4/ik-master.m3u8?tr=sr-360_480_720
Compare this URL to the JSON we had earlier for a similar job with the Transcoder API. The above URL change is far simpler.
When accessed for the first time, ImageKit will automatically start transcoding our video into different resolutions in the background and return the manifest file - ik-master.m3u8
- for HLS streaming. It then stores the manifest file and the various chunks internally.
We can load the above URL directly in an HLS video stream player. That's it! Our adaptive bitrate streaming setup is ready.
We can see in the screenshot below that the browser first started with a couple of 360p video segments for a fast load time before switching up the resolution to 720p because the user's device could load a higher resolution video.
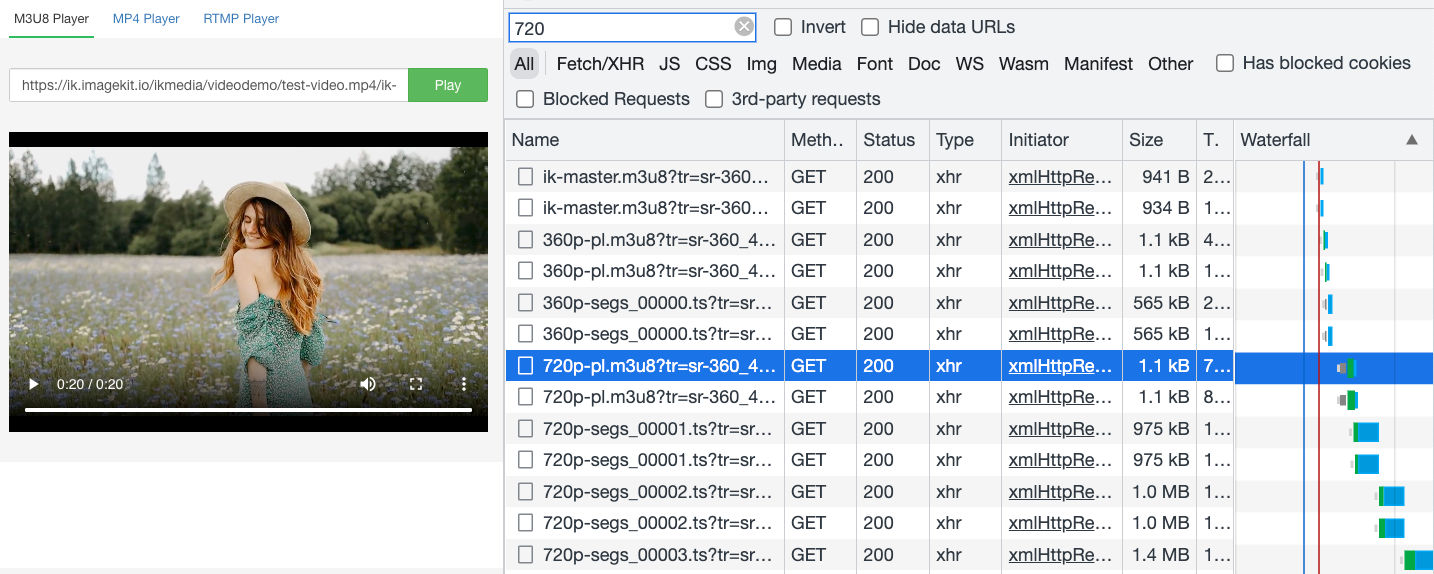
Since video files are large, generating manifest files at multiple resolutions for Adaptive Bitrate Streaming is time-consuming. To improve the development experience and provide certainty about when the asset is ready, ImageKit offers video webhooks. These webhooks allow us to listen to events indicating the completion of this transcoding process. These webhooks are optional, and the process of generating ABS manifests, as explained above, remains the same whether or not we use them.
{
"type": "video.transformation.ready",
"id": "a03031b5-ad5f-4985-8cf5-4de67630f6d7",
"created_at": "2022-06-20T12:00:11.703Z",
"request": {
"x_request_id": "fa98fa2e-d6cd-45b4-acf5-bc1d2bbb8ba9",
"url": "http://ik.imagekit.io/demo/sample-video.mp4?tr=f-webm,q-10",
"user_agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:101.0) Gecko/20100101 Firefox/101.0"
}
....
other fields
....
}
Conclusion
Google Cloud Storage is the object-storage solution provided by Google Cloud. It is very similar to AWS's S3 object storage. We can upload video files to it and load them on the user's device. We can also use Google Cloud's Transcoder API to get ABS for our videos, crop them or watermark them. However, the Transcoder API has a steep learning curve, requires extensive setup, and involves significant effort and costs to re-process a video should our delivery requirements change.
ImageKit is a real-time media optimization, transformation, and management product that simplifies streaming optimized videos directly from your Cloud storage. Once the storage is attached, we can use the URL-based real-time video API to optimize videos automatically, convert them to the right format, adapt them to web and mobile, and build adaptive bitrate streaming using DASH and HLS protocols.
ImageKit offers a great free plan with almost 500 seconds of SD video processing and 20GB of video streaming every month. Sign up now and use ImageKit with Google Cloud Storage for video streaming and optimization on websites and apps.