Firebase Cloud Storage is an excellent cloud service built for app developers who need to store and serve content, such as photos or videos and other files. Once uploaded, these files can be accessed using an SDK and delivered on a website or an app.
Over the past few years, Firebase cloud storage has dramatically simplified app development by providing reliable, secure, and scalable object storage in the cloud.
Video Streaming with Firebase
Firebase is great at storing files and serving them as it is.
One can also deliver videos directly from Firebase storage to the user's device. It will rely on the Range Requests from the client's device and load partial content.
While this is not "streaming" the way Youtube or Netflix stream their videos (using adaptive bitrate streaming techniques), it still does qualify as video streaming, given its definition.
We can use Google Cloud's Video Transcoder API, but the implementation is extensive and requires a deep understanding of video formats, streaming, and encoding. It kind of goes against Firebase's core philosophy of simplifying development for mobile.
For this tutorial, we have a 14.6MB video uploaded to Firebase storage.

We can copy this video's URL and load it directly in the browser or use it in a video
tag as shown below (parts of the URL are masked to prevent direct access).
<video>
<source src="https://firebasestorage.googleapis.com/v0/b/test-firebase-project-xxxxx.appspot.com/o/videos%2Ftest-video.mp4?alt=media&token=044352ec-xxxx-48f9-a93f-a6b034764eac" type="video/mp4" />
</video>
The browser then issues multiple range requests for this video, requesting a shorter video segment. These requests are completed with a 206 status code indicating a partial response from the server (that matches the browser's requested segment).

While this works, there are some shortcomings in load time and user experience.
- Firebase offers no compression of videos. The above video is still as large as what we first uploaded. If we have a 50MB video, it gets delivered as it is to every user, regardless of their device or network conditions resulting in a slow playback experience.
- Firebase storage offers no format conversions. Modern formats like WebM are lighter than MP4, whereas formats like MOV need to be converted to a web-safe format like MP4 for video delivery.
- The above streaming is not Adaptive Bitrate Streaming - the standard for streaming longer videos across devices and network conditions. With Firebase storage alone, there is no easy way to offer Adaptive Bitrate Streaming for our videos.
- One cannot adapt videos for mobile directly from Firebase. Or add watermarks, or create video thumbnails. Without external tools, there is simply no way to transform a video within Firebase. And, even if we opt for third-party tools, integrating them with Firebase can be challenging.
That is where ImageKit, with its real-time video API, comes in. It makes it dead simple to get industry-standard Adaptive bitrate streaming, optimizations, and transformations for our videos in seconds. Let's take a look at how it works.
Using ImageKit for streaming optimized videos from Firebase storage
ImageKit is a real-time media optimization, transformation, and management solution that allows us to stream and optimize videos in near real-time with minimum effort.
ImageKit can be set up to work with any cloud storage in minutes, including Firebase storage, to deliver optimized media in minutes. It uses AWS Cloudfront as a CDN for media delivery.
Therefore, if we use ImageKit for fast media delivery and storage on websites or apps, we won't have to look elsewhere for any third-party tool.
Let's see how we can optimize and stream our test video in our Firebase storage using ImageKit.
You can sign up for a free ImageKit account from here. It offers free 500 seconds of video processing and 20GB of data output every month.
Attaching Firebase storage origin to ImageKit
Attaching Firebase to ImageKit is a straightforward task. We have to add a "Web Server" type of external storage in ImageKit and specify the correct base URL for our storage.
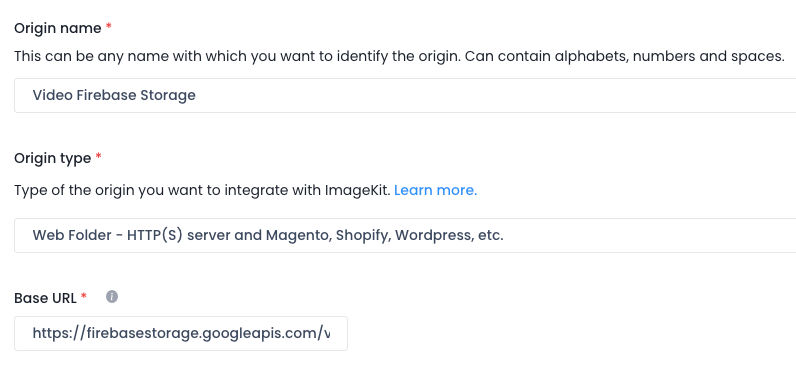
We must then attach this external storage to a URL endpoint in our dashboard, making it accessible via ImageKit. This integration process has been described in detail in this Firebase storage integration document.
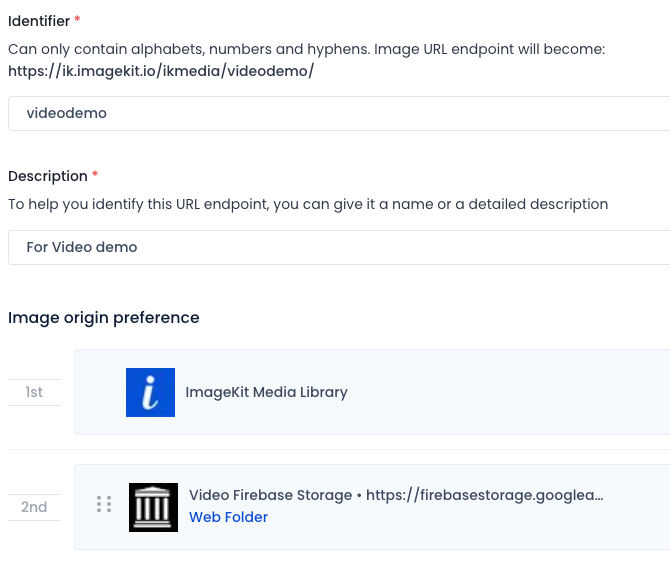
ImageKit does not copy any content from Firebase. Instead, it just accesses the original file when you request it via a URL.
With our storage now attached to ImageKit, let's see how we can optimize the original video for delivery on our user's device.
Streaming optimized video with ImageKit and Firebase Storage
Using the URL below, we can access our video stored in Firebase using ImageKit.
https://ik.imagekit.io/ikmedia/videodemo/o/videos%2Ftest-video.mp4?alt=media&token=044352ec-306d-48f9-a93f-a6b034764eac
Notice that we have just replaced our Firebase base URLhttps://firebasestorage.googleapis.com/v0/b/test-firebase-project-xxxxx.appspot.com
with the ImageKit URL endpointhttps://ik.imagekit.io/ikmedia/videodemo/
, and the query parameters and the rest of the path are the same.
When requested using this URL, ImageKit automatically identifies the requesting device and its video format support. This information converts the video to WebM or MP4, the two most widely used web-safe video formats.
It also compresses the video using format-specific compression algorithms to decrease the video file size without significantly reducing the visual quality.
Unlike other third-party tools, these video optimizations are accessible in the ImageKit dashboard and require no extensive setup or video encoding knowledge.
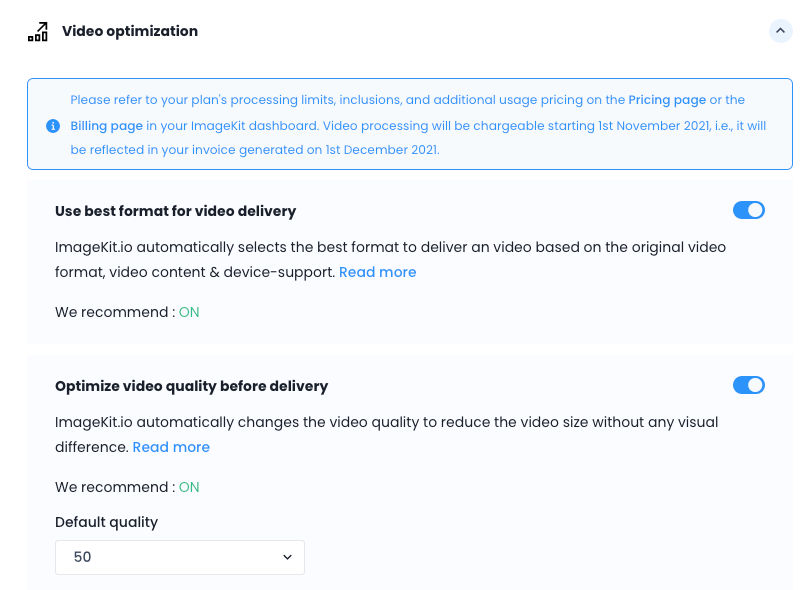
When we deliver our sample video via ImageKit, its size drops from 14.7MB to just 9.1MB when delivered in WebM format on Chrome.
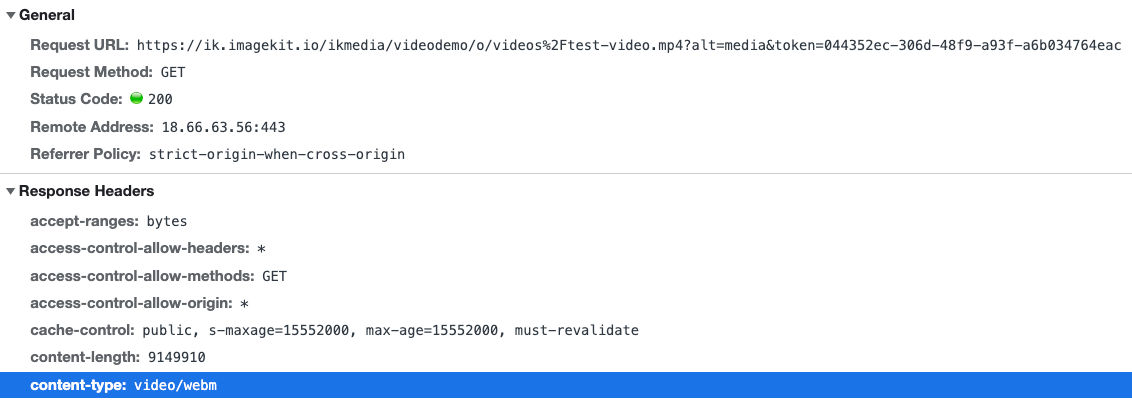
This is a 40% improvement in the video streaming experience we can offer with Firebase storage just by using ImageKit. And with the integrated CDN in ImageKit, the video load time is also super fast.
Transforming videos for different use cases with ImageKit
ImageKit offers a unique URL-based real-time video API. This API makes it easy to adapt videos to different devices and placeholders, and create video snippets and thumbnails, to create a complete video experience without using any specialized video player on the client's device. All the transformations are real-time and require no external setup or extensive coding.
To resize our video to a 200px width, we have to add the video transformation parameter tr=w-200
to our URL, as shown below.
https://ik.imagekit.io/ikmedia/videodemo/o/videos%2Ftest-video.mp4?tr=w-200&alt=media&token=044352ec-306d-48f9-a93f-a6b034764eac
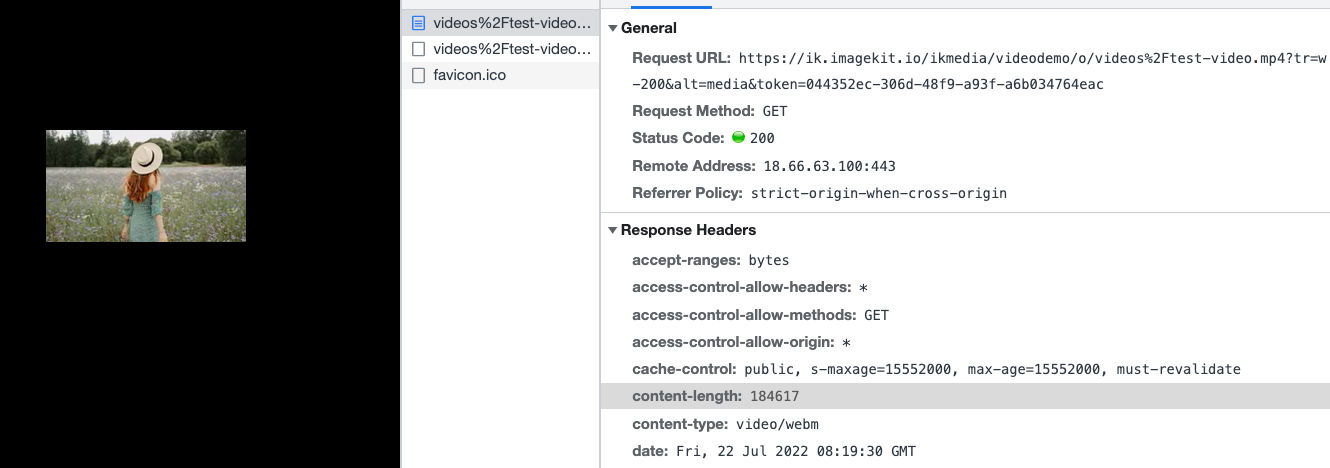
ImageKit automatically converts the video to WebM format (the content-type
header in the screenshot above is video/webm
), and the video size drops to just 184KB.
We can also convert our video to a vertical video to adapt to platforms like Instagram reels by specifying the appropriate height and width transformations directly in the video URL. ImageKit takes care of cropping the video and returns the size we requested. For a 400 x 640 vertical video requested using the URL below, we get the video size at 2.3MB.
https://ik.imagekit.io/ikmedia/videodemo/o/videos%2Ftest-video.mp4?tr=w-400,h-640&alt=media&token=044352ec-306d-48f9-a93f-a6b034764eac
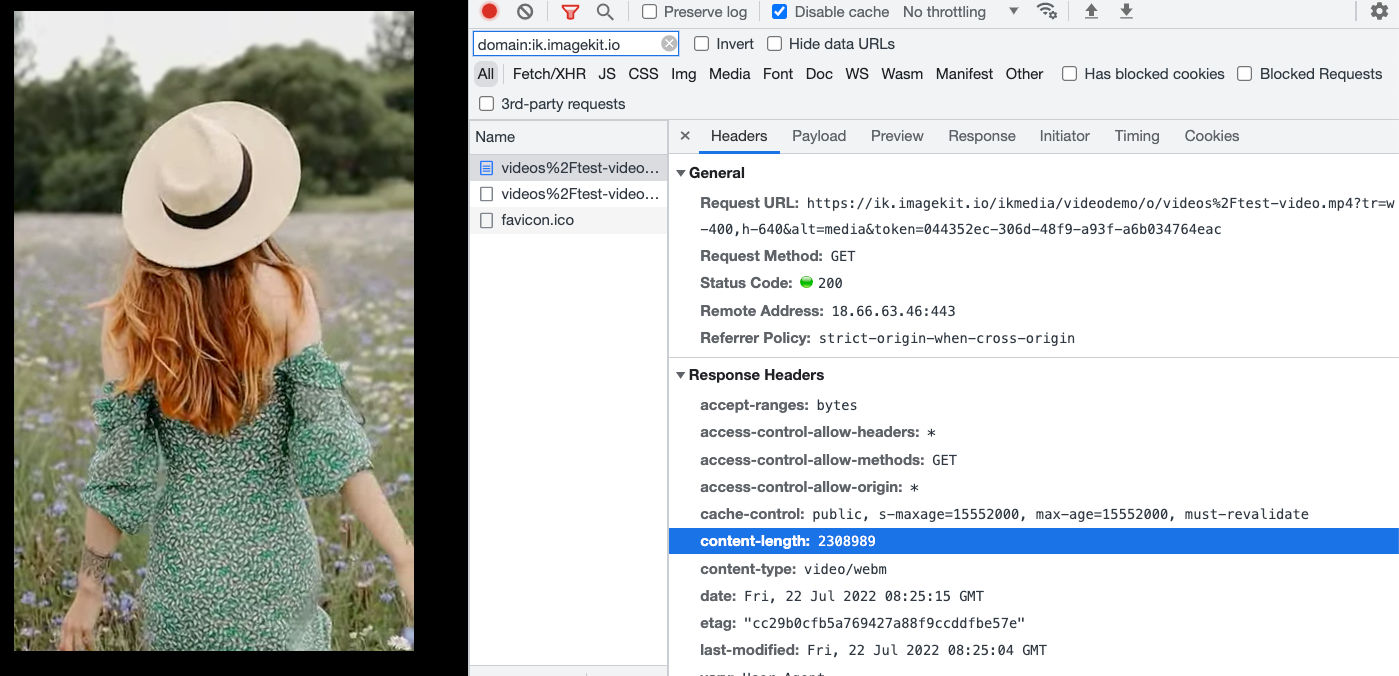
We can also watermark our video and control how the watermark gets placed in real-time using the URL-based video transformation parameters.
https://ik.imagekit.io/ikmedia/videodemo/o/videos%2Ftest-video.mp4?tr=w-400,h-640,l-image,i-logo_HuFO6vJ2x.png,lx-10,ly-10,w-200,l-end&alt=media&token=044352ec-306d-48f9-a93f-a6b034764eac
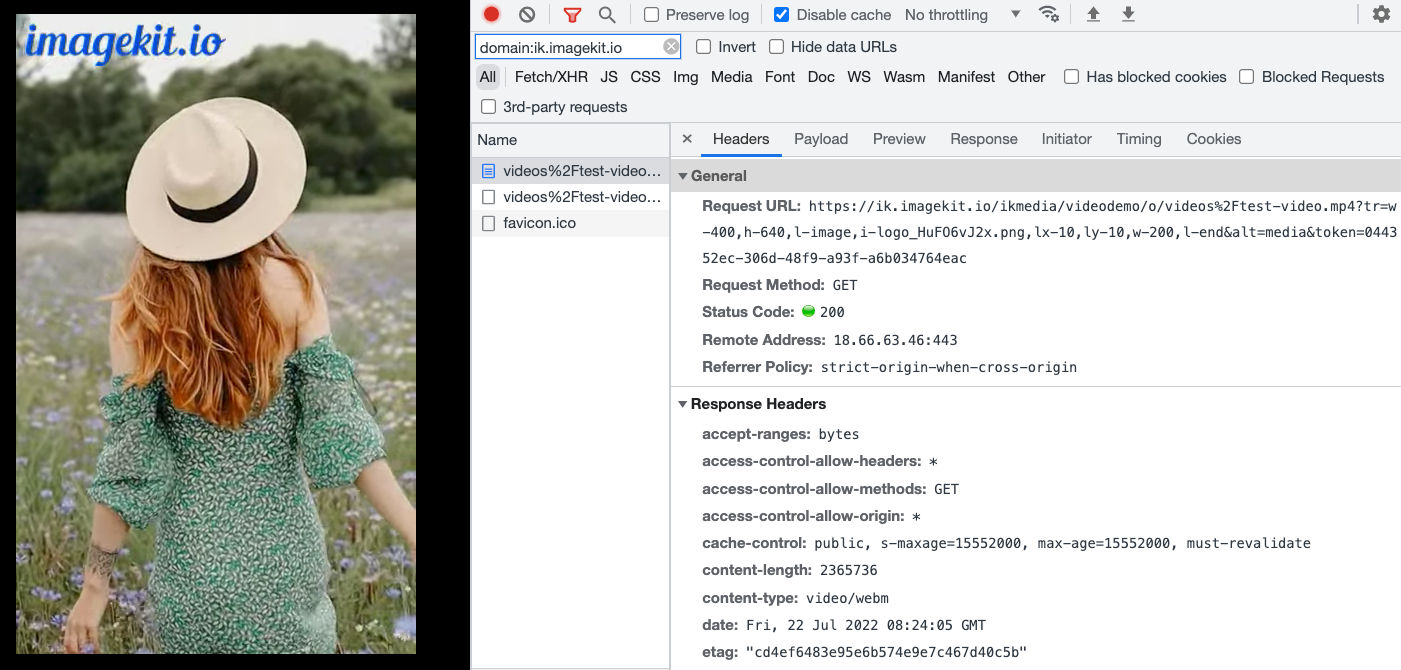
Adaptive bitrate streaming for videos with Firebase and ImageKit
Adaptive bitrate streaming helps us adjust the video quality or resolution if the user's network speed or device changes. Streaming services such as Netflix and Youtube extensively use this, and most of us would have experienced this adjustment in quality ourselves.
ImageKit makes it super simple to use DASH and HLS - the two most popular Adaptive Bitrate Streaming protocols with our videos hosted on Firebase Storage. Unlike the processing offered by Google Transcoding APIs, we can implement ImageKit's URL-based Adaptive Bitrate Streaming API in seconds, and in real-time, by making small URL changes.
For example, we can get an HLS stream ready at 360p, 480p, and 720p resolutions by modifying our video URL as shown below.
https://ik.imagekit.io/ikmedia/videodemo/o/videos%2Ftest-video.mp4/ik-master.m3u8?tr=sr-360_480_720&alt=media&token=044352ec-306d-48f9-a93f-a6b034764eac
When accessed for the first time, ImageKit will automatically start transcoding our video into different resolutions in the background and return the manifest file - ik-master.m3u8
- for HLS streaming.
We can load the above URL directly in a HLS video stream player. That's it! Our adaptive bitrate streaming setup is ready.
We can see in the screenshot below that the browser first started with a couple of 360p video segments for a fast load time before switching up the resolution to 720p because the user's device could load a higher resolution video.
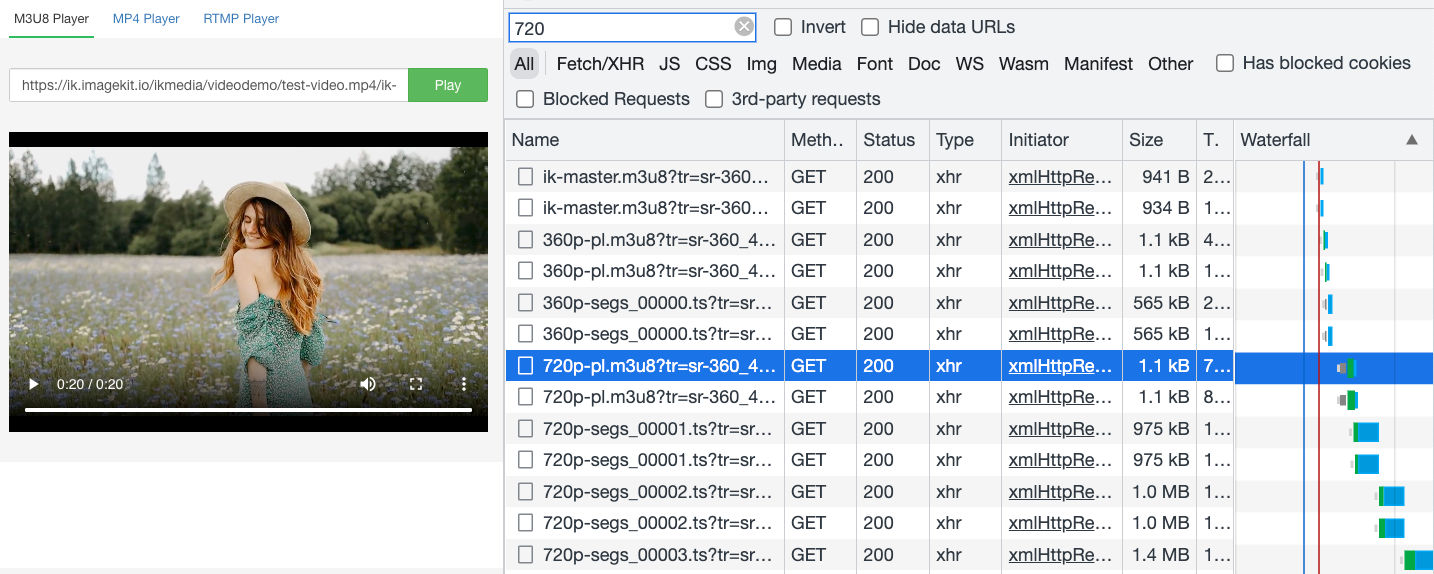
Generating manifest files at multiple resolutions for Adaptive Bitrate Streaming is time-consuming. To improve the development experience and provide certainty as to when the asset is ready, ImageKit offers video webhooks. These webhooks allow us to listen to events indicating the completion of this transcoding process. These webhooks are optional, and the process of generating ABS manifests, as explained above, remains the same whether or not we use them.
{
"type": "video.transformation.ready",
"id": "a03031b5-ad5f-4985-8cf5-4de67630f6d7",
"created_at": "2022-06-20T12:00:11.703Z",
"request": {
"x_request_id": "fa98fa2e-d6cd-45b4-acf5-bc1d2bbb8ba9",
"url": "http://ik.imagekit.io/demo/sample-video.mp4?tr=f-webm,q-10",
"user_agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:101.0) Gecko/20100101 Firefox/101.0"
}
....
other fields
....
}
Conclusion
Firebase storage is a widely popular, mobile developer-friendly object storage. We can upload video files to it and load them on the user's device. However, without any other feature for video optimization, streaming, or transformations, it leaves much to be desired.
ImageKit is a real-time media optimization and transformation service that simplifies streaming optimized videos directly from our Firebase storage. Once the storage is attached, we can use the URL-based real-time video API to optimize videos automatically, adapt them to different devices, and deliver adaptive bitrate streams using DASH and HLS protocols.
ImageKit offers a decent free plan with almost 500 seconds of SD video processing and 20GB of video streaming every month. Sign up now and use ImageKit with Firebase for video streaming on websites and apps.