If your image doesn’t fit the layout, you can resize it in the HTML. One of the simplest ways to resize an image in the HTML is using the height
and width
attributes on the img
tag. These values specify the height and width of the image element. The values are set in px i.e. CSS pixels.
For example, the original image is 640×960.
https://ik.imagekit.io/ikmedia/women-dress-2.jpg
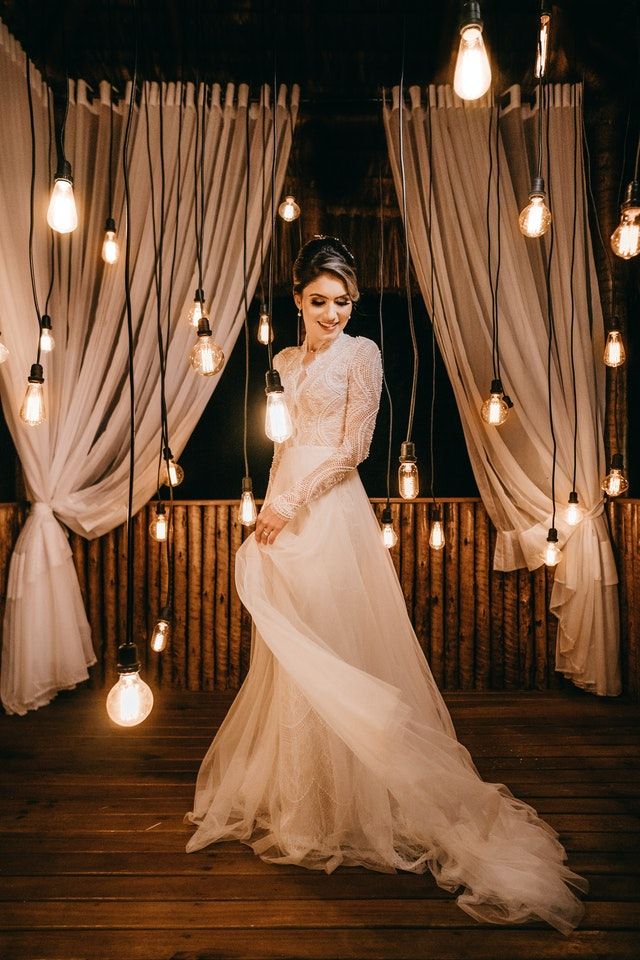
We can render it with a height of 500 pixels and a width of 400 pixels
<img src="https://ik.imagekit.io/ikmedia/women-dress-2.jpg"
width="400"
height="500" />
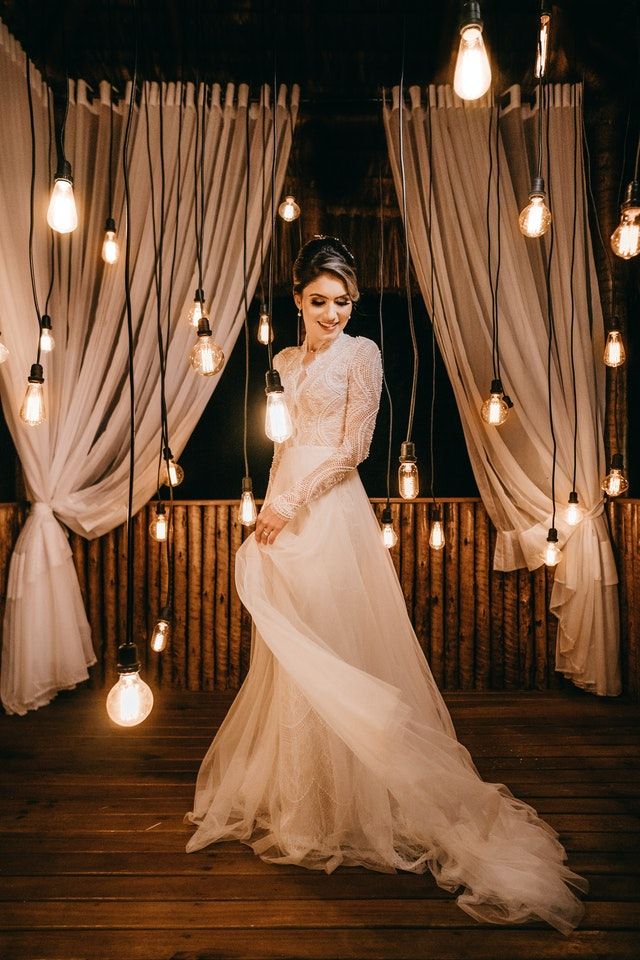
If the image element's required height and width don’t match the image's actual dimensions, then the browser downscales (or upscale) the image. The exact algorithm used by the browser for scaling can vary and depends on the underlying hardware and OS.
There are a couple of downsides of client-side image resizing, mainly poor image quality and slower image rendering. To overcome this, you should serve already resized images from the server. You can use Thumbor or a free image CDN like ImageKit.io to resize images dynamically using URL parameters.
Resizing an image in CSS
You can also specify the height and width in CSS.
img {
width: 400px,
height: 300px
}
Preserving the aspect ratio while resizing images
When you specify both height
and width
, the image might lose its aspect ratio. You can preserve the aspect ratio by specifying only width
and setting height
to auto
using CSS property.
img {
width: 400px,
height: auto
}
This will render a 400px wide image. The height is adjusted accordingly to preserve the aspect ratio of the original image. You can also specify the height
attribute and set width
as auto
, but most layouts are generally width constrained and not height.
Responsive image which adjusts based on available width
You can specify the width in percentage instead of an absolute number to make it responsive. By setting width
to 100%
, the image will scale up if required to match the parent element’s width. It might result in a blurred image as the image can be scaled up to be larger than its original size.
img {
width: 100%;
height: auto;
}
Alternatively, you can use the max-width
property. By setting
max-width:100%;
the image will scale down if it has to, but never scale up to be larger than its original size.
img {
max-width: 100%;
height: auto;
}
How to resize & crop image to fit an element area?
So far, we have discussed how to resize an image by specifying height
or width
or both of them.
When you specify both height
and width
, the image is forced to fit the requested dimension. It could change the original aspect ratio. At times, you want to preserve the aspect ratio while the image covers the whole area even if some part of the image is cropped. To achieve this, you can use:
- Background image
object-fit
css property
Resizing background image
background-image
is a very powerful CSS property that allows you to insert images on elements other than img
. You can control the resizing and cropping of the image using the following CSS attributes-
background-size
- Size of the imagebackground-position
- Starting position of a background image
background-size
By default, the background image is rendered at its original full size. You can override this by setting the height and width using the background-size
CSS property. You can scale the image upward or downward as you wish.
<style>
.background {
background-image: url("/image.jpg");
background-size: 150px;
width: 300px;
height: 300px;
border: solid 2px red;
}
</style>
<div class="background">
</div>
Possible values of background-size
:
auto
- Renders the image at full sizelength
- Sets the width and height of the background image. The first value sets the width, and the second value sets the height. If only one value is given, the second is set toauto
. For example,100px 100px
or50px
.percentage
- Sets the width and height of the background image in percent of the parent element. The first value sets the width, and the second value sets the height. If only one value is given, the second is set toauto
. For example,100% 100%
or50%
.
It also has 2 special values contain
and cover
:
background-size:containscontains
- It preserves the original aspect ratio of the image, but the image is resized so that it is fully visible. The longest of either the height or width will fit in the given dimensions, regardless of the size of the containing box.
<style>
.background {
background-image: url("/image.jpg");
background-size: contains;
width: 300px;
height: 300px;
border: solid 2px red;
}
</style>
<div class="background">
</div>
background-size:covercover
- It preserves the original aspect ratio but resizes the image to cover the entire container, even if it has to upscale the image or crop it.
<style>
.background {
background-image: url("/image.jpg");
background-size: cover;
width: 300px;
height: 300px;
border: solid 2px red;
}
</style>
<div class="background">
</div>
object-fit CSS property
You can use the object-fit
CSS property on the img
element to specify how the image should be resized & cropped to fit the container. Before this CSS property was introduced, we had to resort to using a background image.
Along with inherit
, initial
, and unset
, there are 5 more possible values for object-fit:
contain
: It preserves the original aspect ratio of the image, but the image is resized so that it is fully visible. The longest of either the height or width will fit in the given dimensions, regardless of the size of the containing box.cover
: It preserves the original aspect ratio but resizes the image to cover the entire container, even if it has to upscale the image or crop it.fill
: This is the default value. The image will fill its given area, even if it means losing its aspect ratio.none
: The image is not resized at all, and the original image size fills the given area.scale-down
: The smaller of either contain or none.
You can use object-position
to control the starting position of the image in case a cropped part of the image is being rendered.
Let’s understand these with examples.
The following image’s original width is 1280px and height is 854px. Here it is stretching to maximum available width using max-width: 100%
.
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="max-width: 100%;" />
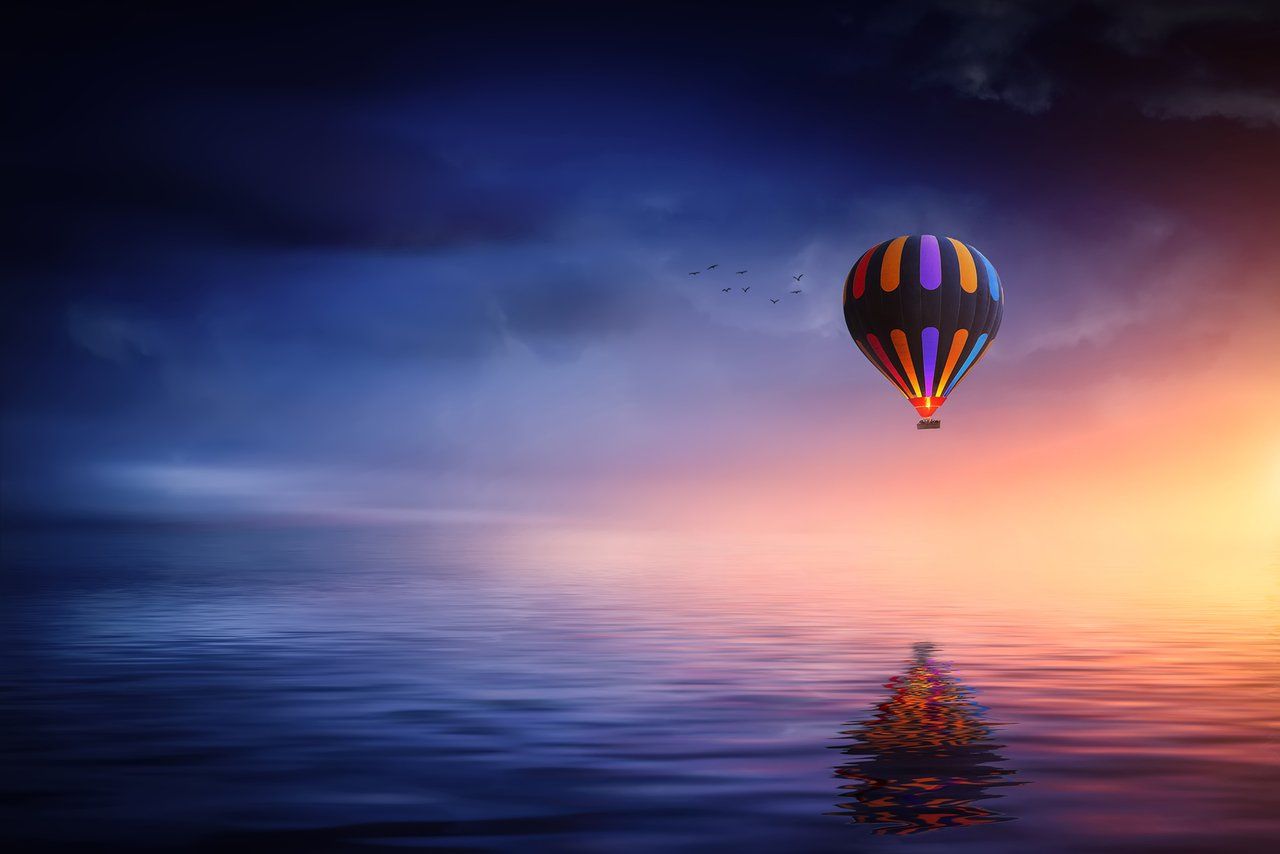
object-fit:contains
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:contain;
width:200px;
height:300px;
border: solid 1px #CCC"/>
The original aspect ratio of the image is same, but the image is resized so that it is fully visible. We have added 1px
border around the image to showcase this.
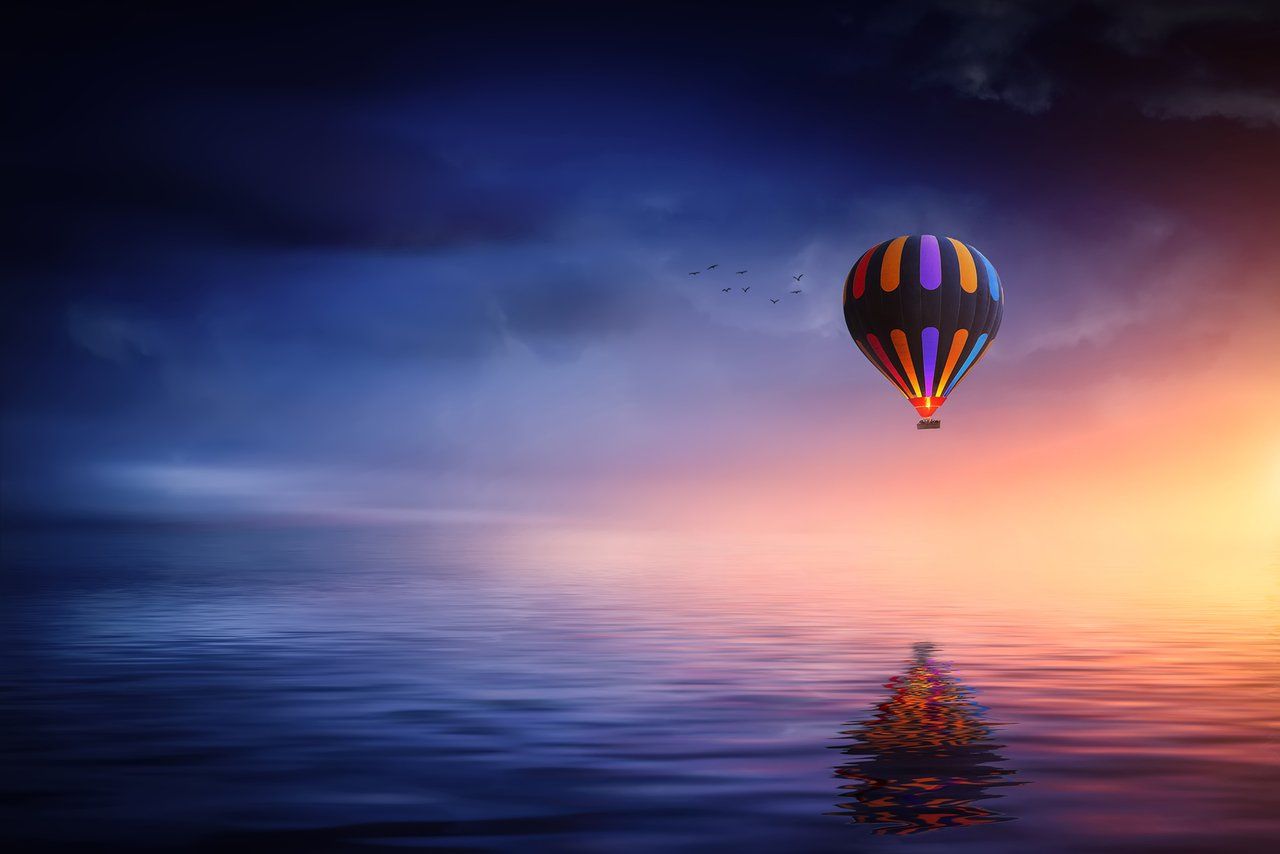
object-fit:cover
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:cover;
width:200px;
height:300px;
border: solid 1px #CCC"/>
The original aspect ratio is preserved but to cover the whole area image is clipped from the left and right side.
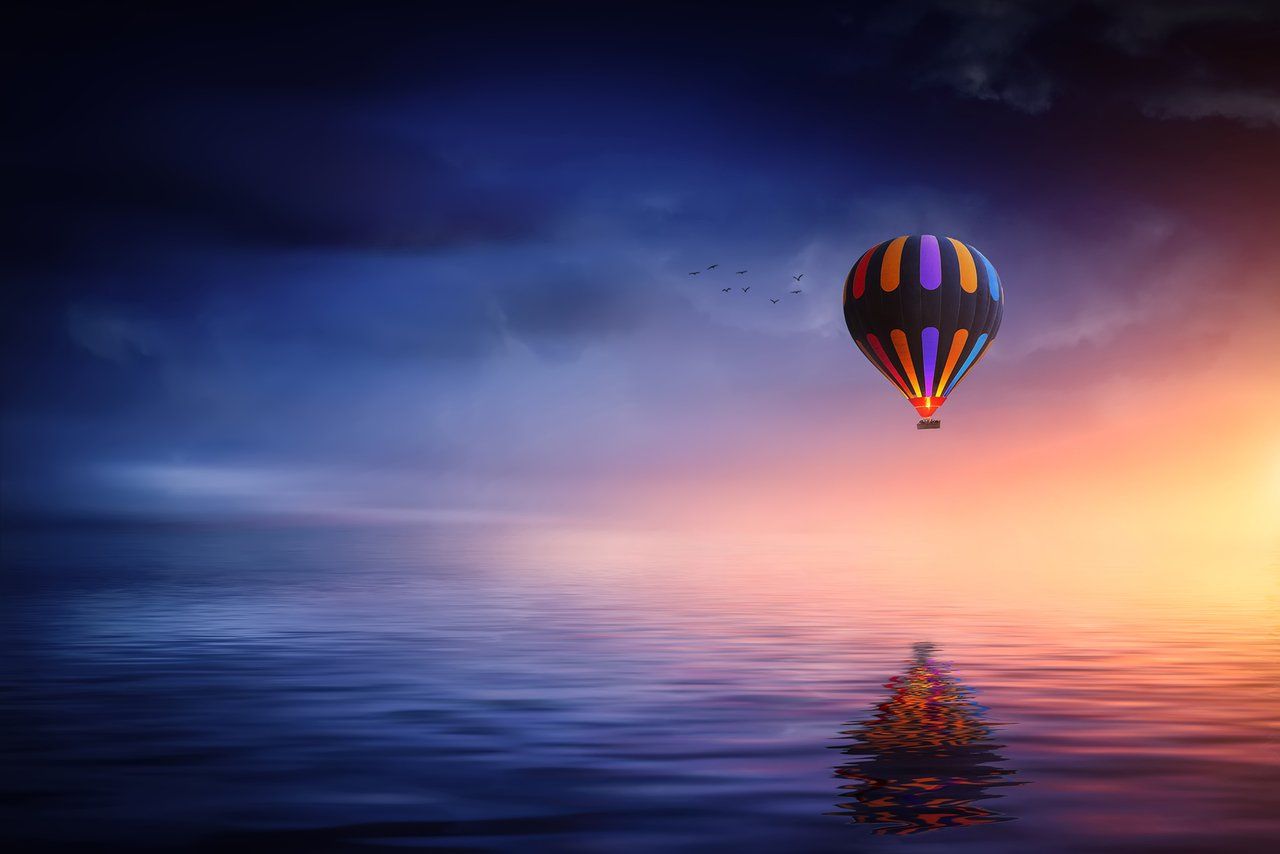
object-fit:fill
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:fill;
width:200px;
height:300px;
border: solid 1px #CCC"/>
Image is forced to fit into a 200px wide container with height 300px, the original aspect ratio is not preserved.
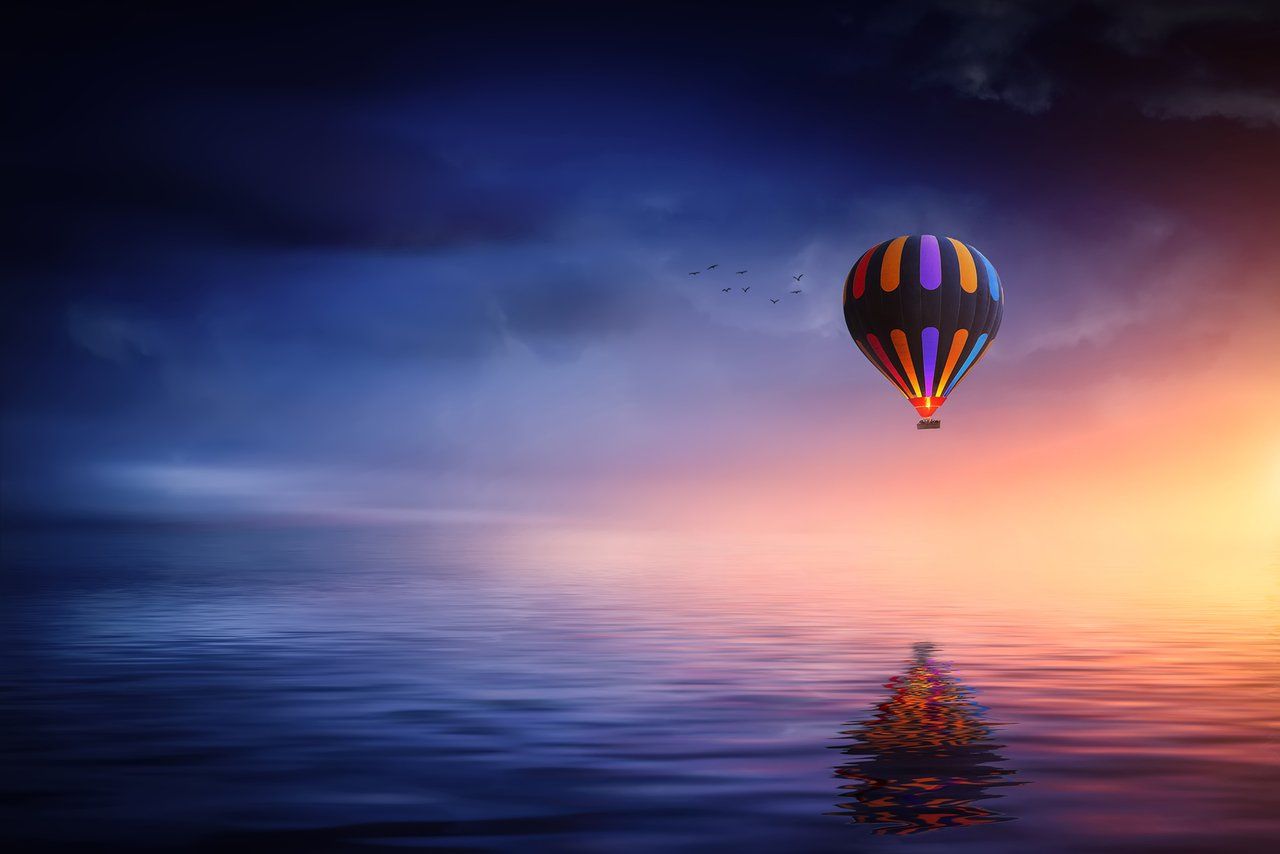
object-fit:none
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:none;
width:200px;
height:300px;
border: solid 1px #CCC"/>
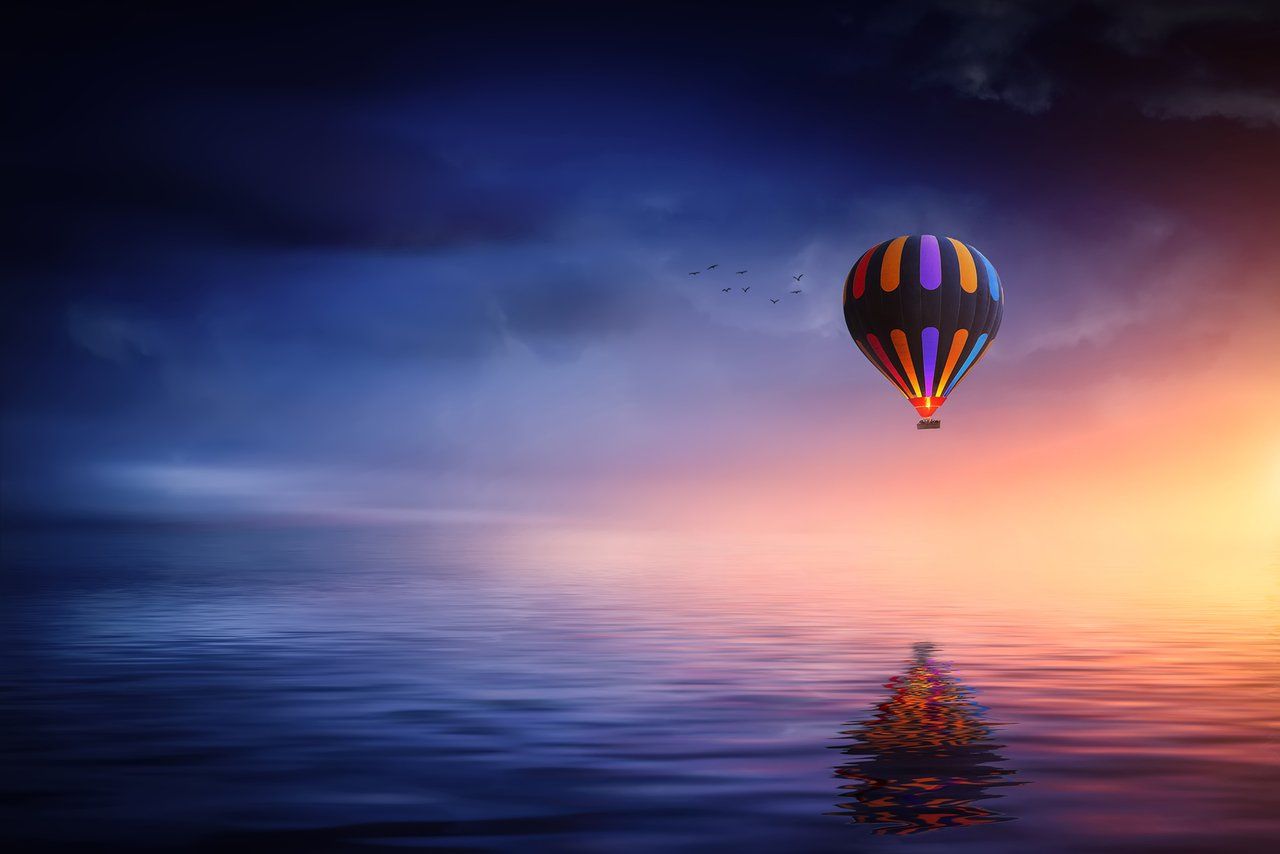
object-fit:scale-down
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:scale-down;
width:200px;
height:300px;
border: solid 1px #CCC"/>
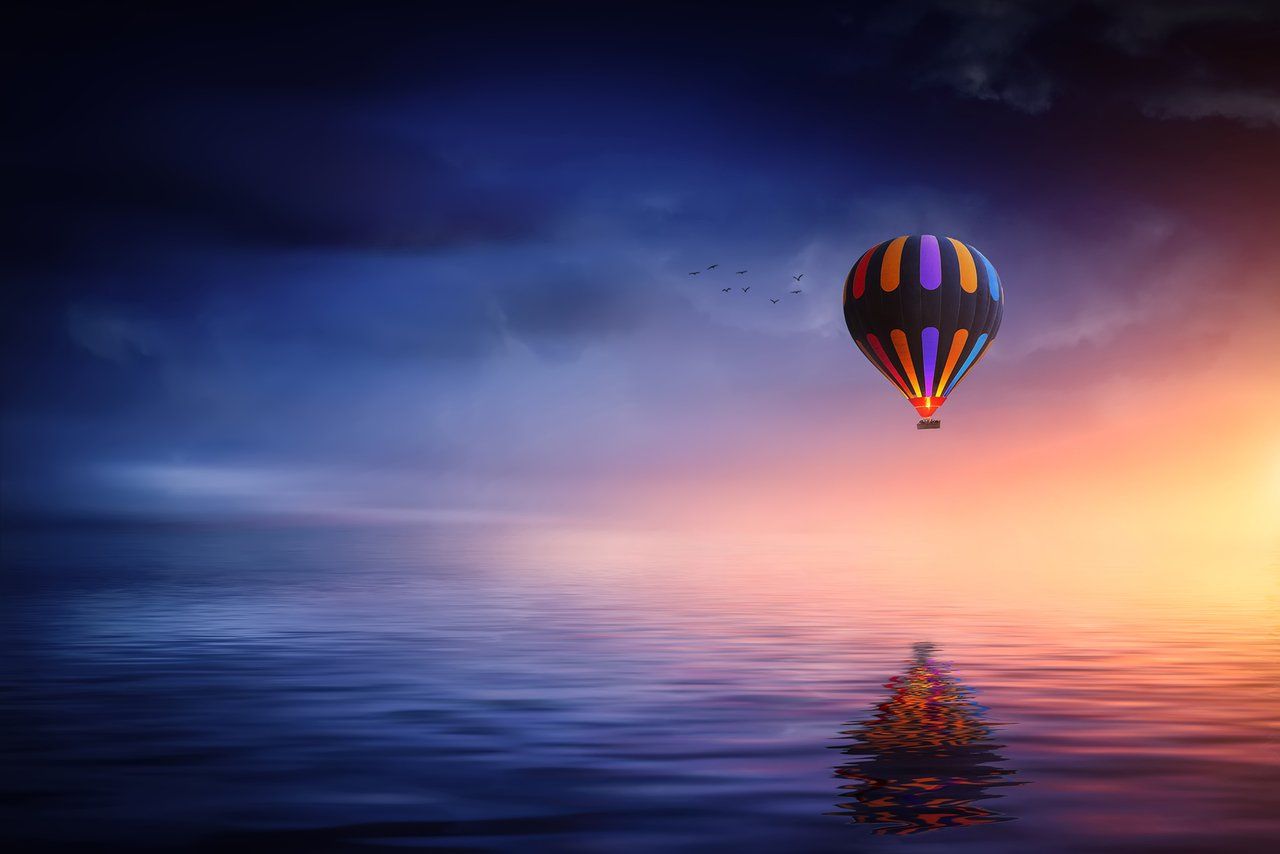
object-fit:cover and object-position:right
<img src="https://ik.imagekit.io/ikmedia/backlit.jpg"
style="object-fit:cover;
object-position: right;
width:200px;
height:300px;
border: solid 1px #CCC"/>
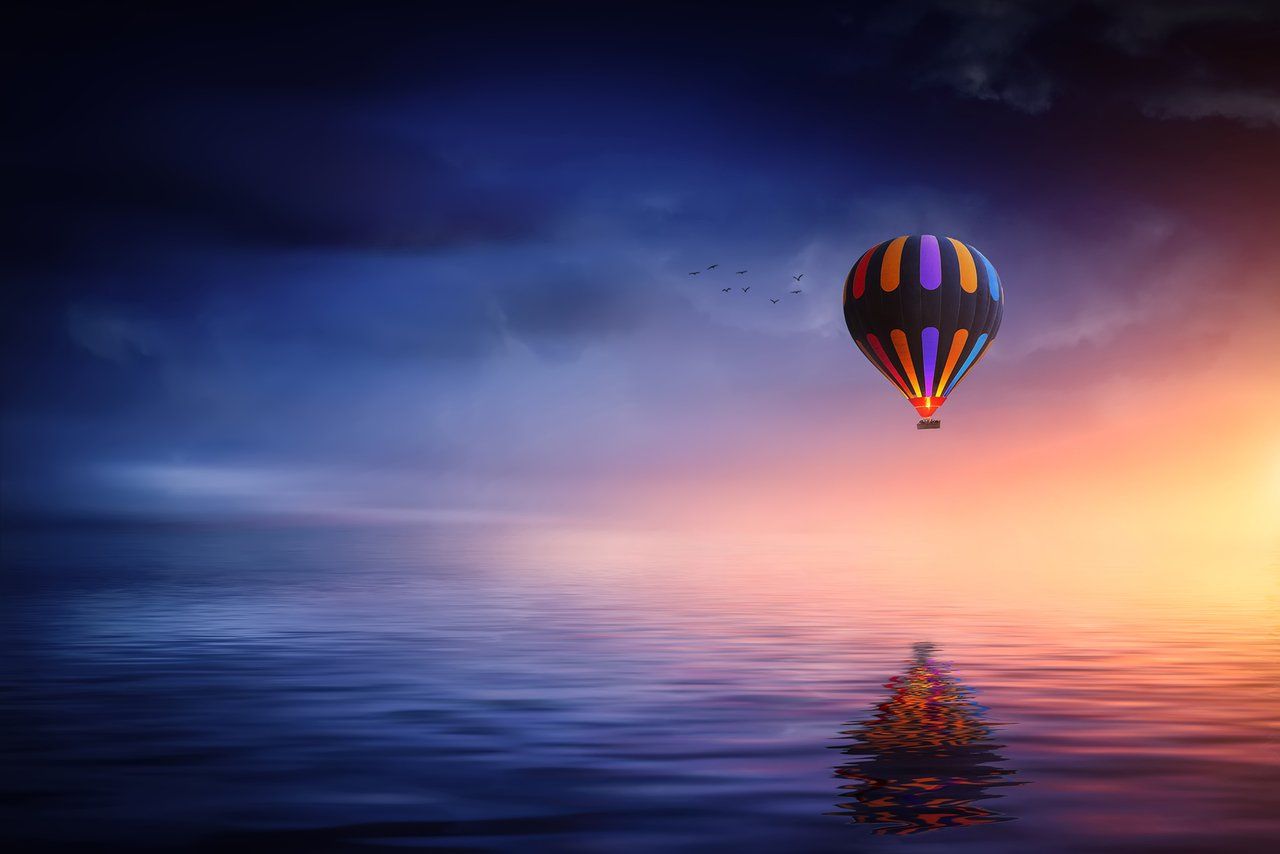
Downsides of client-side image resizing
There are certain downsides of client-side resizing that you should keep in mind.
1. Slow image rendering
Since the full-sized image is loaded anyway before resizing happens in the browser, it takes more time to finish downloading and finally rendering. This means that if you have a large, 1.5 megabyte, 1024×682 photograph that you are displaying at 400px in width, the whole 1.5-megabyte image is downloaded by the visitor before the browser resizes it down to 400px.
You can see this download time on the network panel, as shown in the screenshot below.
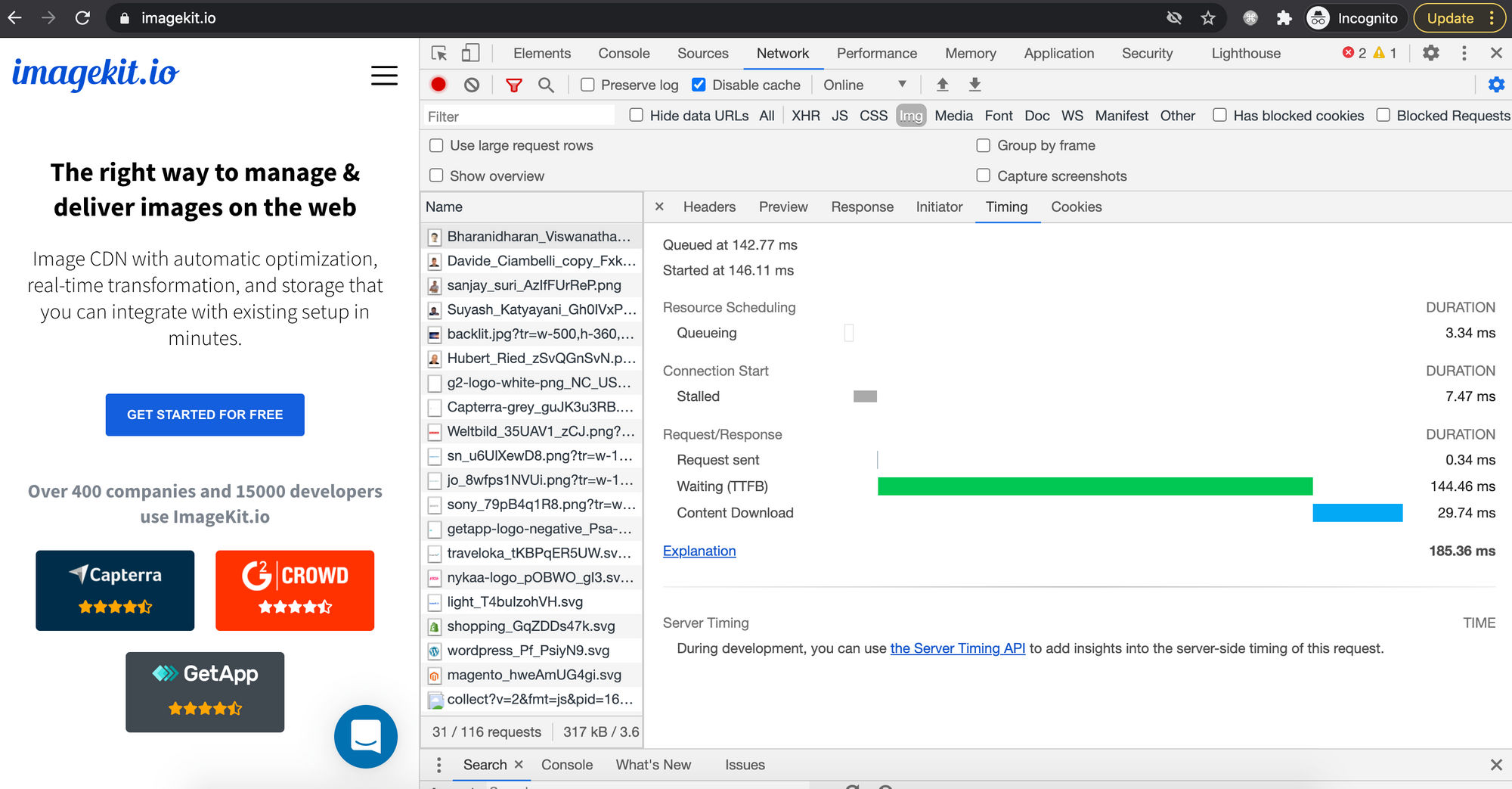
On the other hand, if you resize the image on the server using some program or an image CDN, then the browser doesn't have to load a large amount of data and waste time decoding & rendering it.
With ImageKit.io, you can easily resize an image using URL parameters. For example -
Original image
https://ik.imagekit.io/ikmedia/women-dress-2.jpg
400px wide image with aspect ratio preserved
https://ik.imagekit.io/ikmedia/women-dress-2.jpg?tr=w-400
2. Poor image quality
The exact scaling algorithm used by the browser can vary, and its performance depends upon underlying hardware and OS. When a relatively bigger image is resized to fit a smaller container, the final image could be noticeably blurry.
There is a tradeoff between speed and quality. The final choice depends upon the browser. Firefox 3.0 and later versions use a bilinear resampling algorithm, which is tuned for high quality rather than speed. But this could vary.
You can use the image-rendering
CSS property, which defines how the browser should render an image if it is scaled up or down from its original dimensions.
/* Keyword values */
image-rendering: auto;
image-rendering: crisp-edges;
image-rendering: pixelated;
/* Global values */
image-rendering: inherit;
image-rendering: initial;
image-rendering: unset;
3. Bandwidth wastage
Since the full-sized image is being loaded anyway, it results in wastage of bandwidth, which could have been saved. Data transfer is not cheap. In addition to increasing your bandwidth bills, it also costs your users real money.
If you are using an image CDN, you can further reduce your bandwidth consumption by serving images in next-gen formats e.g. WebP or AVIF.
The user friendly dashboard will also show you how much bandwidth you have saved so far
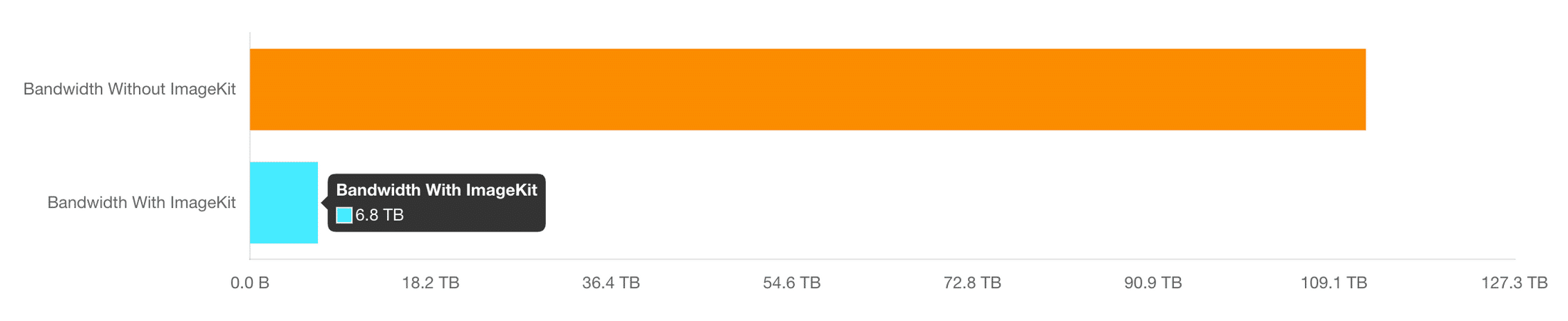
4. Increased memory and processing requirements on client devices
Resizing large images to fit a smaller container is expensive and can be painful on low-end devices where both memory and processing power is limited. This slows down the whole web page and degrades the user experience.
Summary
When implementing web pages, images need to fit the layout perfectly. Here is what you need to remember to be able to implement responsive designs:
- Avoid client-side (browser) resizing at all if you can. This means serve correctly sized images from the server. It results in less bandwidth usage, faster image loading, and higher image quality. There are many open-source image processing libraries if you want to implement it yourself. Or better, you can use a free image CDN which will provide all these features and much more with a few lines of code.
- Never upscale a raster image i.e. JPEG, PNG, WebP, or AVIF images, should never be upscaled as it will result in a blurred output.
- You should use the SVG format for icons and graphics if required in multiple dimensions in the design.
- While resizing, if you want to preserve the aspect ratio of original images - Only specify one of
width
andheight
and set the other toauto
. - If you want the image to fit the entire container while preserving the aspect ratio, even if some part is cropped or the image is upscaled - Use the
object-fit
CSS property or set a background image using thebackground-image
CSS property. - Control the starting position of the image using
object-position
while usingobject-fit
. In background images, usebackground-position
.